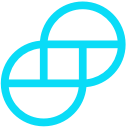
Gemini
CryptocurrencyUsing the Gemini REST API with JavaScript
The Gemini REST API is a powerful tool for building custom trading tools and applications, providing access to a range of features including order management, account balances, and historical data. In this brief guide, we'll explore how to use this API with JavaScript.
Creating a Gemini API Key
Before we can use the Gemini REST API, we must first create an API key on the Gemini website. To do this, follow these steps:
- Log in to your Gemini account and navigate to the API tab.
- Click "Generate New API Key" and follow the prompts.
- Once your key has been generated, copy the
API Key
andAPI Secret
for use in future requests.
Making a Simple API Request
To make a request to the Gemini REST API, we'll use the popular axios
library. Here's an example of how to request the Gemini ticker data:
const axios = require('axios');
const API_KEY = 'your_api_key';
const API_SECRET = 'your_api_secret';
const GEMINI_API_URL = 'https://api.gemini.com/v1';
axios({
method: 'GET',
url: `${GEMINI_API_URL}/pubticker/btcusd`
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
In this example, we simply import the axios
library and set our API key and secret. We then make a GET
request to the pubticker/btcusd
endpoint and print the response data to the console.
Example: Creating a New Order
Let's look at a more complex example, creating a new order on the Gemini exchange. To keep things simple, we'll create a basic createOrder
function that takes in the required parameters and returns a Promise that resolves with the API response.
const querystring = require('querystring');
const crypto = require('crypto');
const axios = require('axios');
const API_KEY = 'your_api_key';
const API_SECRET = 'your_api_secret';
const GEMINI_API_URL = 'https://api.gemini.com/v1';
function createOrder(symbol, amount, price, side, options) {
// Set required parameters.
const options = options || {};
const nonce = Date.now();
const payload = {
request: `/v1/order/new`,
nonce,
symbol,
amount: amount.toString(),
price: price.toString(),
side: side.toLowerCase(),
type: 'exchange limit',
client_order_id: nonce.toString(),
...options,
};
// Sign the payload.
const signature = crypto
.createHmac('sha384', API_SECRET)
.update(Buffer.from(JSON.stringify(payload)))
.digest('hex');
// Set the request headers.
const headers = {
'Content-Type': 'text/plain',
'Content-Length': '0',
'X-GEMINI-APIKEY': API_KEY,
'X-GEMINI-PAYLOAD': Buffer.from(JSON.stringify(payload)).toString('base64'),
'X-GEMINI-SIGNATURE': signature,
'Cache-Control': 'no-cache',
};
// Make the request.
return axios({
method: 'POST',
url: `${GEMINI_API_URL}/order/new`,
headers,
data: querystring.stringify(payload),
});
}
createOrder('btcusd', 1, 40000, 'buy')
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
In this example, we define a basic createOrder
function that takes in the required parameters and a set of optional options
. We first set the required parameters, including a unique nonce
value that is used to ensure request uniqueness and to prevent replay attacks. We then sign the payload using our API secret, set the request headers, and make the POST
request to the order/new
endpoint.
Conclusion
In this brief guide, we've explored how to use the Gemini REST API with JavaScript. By leveraging popular libraries like axios
and crypto
, we can create a range of custom trading tools and applications that interact with the Gemini exchange. Remember to always follow best practices when handling sensitive data, such as keeping your API key and secret secure and properly managing nonce values to prevent replay attacks.