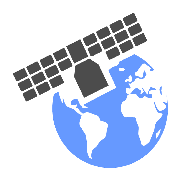
GeoJS
GeocodingWorking with the GeoJS Public API
If you're working with location data, GeoJS has a public API that makes it easy to retrieve a variety of location-based information. In this post, we'll explore the GeoJS public API and walk through some example code in JavaScript.
Retrieving IP-based Location Information
If you have a user's IP address, you can use the GeoJS API to retrieve location information about them. Here's an example code snippet:
const ipAddress = "123.45.67.89";
const url = `https://get.geojs.io/v1/ip/geo/${ipAddress}.json`;
fetch(url)
.then((response) => response.json())
.then((data) => {
console.log(data);
});
In this code, we're using the fetch()
function to send an HTTP request to the GeoJS API endpoint for IP-based location information. We're passing in the IP address as part of the URL, and we're specifying that we want to receive the response in JSON format. Once we get the response, we log the data to the console.
The response we get should look something like this:
{
"ip": "123.45.67.89",
"country": "United States",
"region": "California",
"city": "Mountain View",
"latitude": 37.4192,
"longitude": -122.0574
}
Retrieving Geolocation Information for a Specific IP Address
If you have a specific IP address that you want to retrieve location information for, you can use the GeoJS API endpoint for that purpose. Here's an example code snippet:
const ipAddress = "123.45.67.89";
const url = `https://get.geojs.io/v1/ip/geo/${ipAddress}.json`;
fetch(url)
.then((response) => response.json())
.then((data) => {
const lat = data.latitude;
const lng = data.longitude;
const coords = `${lat},${lng}`;
const url = `https://get.geojs.io/v1/reverse/coords/${coords}/short.json`;
fetch(url)
.then((response) => response.json())
.then((data) => {
console.log(data);
});
});
In this code, we're first retrieving the geolocation information for a specific IP address, just like in the previous example. We then extract the latitude and longitude values from the response and use them to construct a new URL for retrieving the reverse geolocation information. Once we get the response, we log the data to the console.
The response we get should look something like this:
{
"city": "Mountain View",
"region": "California",
"country": "US"
}
Retrieving information for a list of IP Addresses
If you need to retrieve location information for a list of IP addresses, you can use the GeoJS API batch endpoint. Here's an example code snippet:
const ipAddresses = ["123.45.67.89", "98.76.54.32"];
const url = `https://get.geojs.io/v1/ip/geo-batch/${ipAddresses.join(",")}.json`;
fetch(url)
.then((response) => response.json())
.then((data) => {
console.log(data);
});
In this code, we're using the GeoJS API batch endpoint to retrieve location information for a list of IP addresses. We pass the IP addresses as a comma-separated list in the URL. Once we get the response, we log the data to the console.
The response we get should look something like this:
[
{
"ip": "123.45.67.89",
"country": "United States",
"region": "California",
"city": "Mountain View",
"latitude": 37.4192,
"longitude": -122.0574
},
{
"ip": "98.76.54.32",
"country": "United States",
"region": "New York",
"city": "New York City",
"latitude": 40.7857,
"longitude": -73.9723
}
]
Conclusion
The GeoJS public API is a powerful tool for working with location-based data. With the examples we've covered in this post, you have a solid starting point for using the API in your own JavaScript projects.