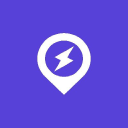
Geocodify
GeocodingGeocodify API Documentation
Geocodify provides a public API to enable developers to easily access geocoding services. The API is well-documented and can be accessed using HTTP/REST requests. This article gives a brief overview of the Geocodify API documentation and provides some example API requests using JavaScript.
API Documentation Overview
The Geocodify API documentation consists of many endpoints that can be used to perform geocoding operations. These endpoints provide various ways to search for an address, or reverse geocode a coordinate. Here are some of the main endpoints for using the Geocodify API:
-
Forward geocoding: This endpoint allows users to search for the geographic coordinates of an address by passing an address string as a parameter.
-
Reverse geocoding: This endpoint enables users to get the nearest street address and other location details by passing in geographic coordinates as input.
-
Batch geocoding: This endpoint allows users to submit multiple forward or reverse geocoding requests at the same time.
-
Autocomplete: This endpoint provides auto-suggestions of places or addresses as users type a query.
Example API Requests Using JavaScript
Here are some examples of how to perform HTTP requests using JavaScript to access the Geocodify API:
Forward Geocoding Request
const address = '123 Main St, New York, NY';
const key = 'your_geocodify_api_key';
fetch('https://api.geocodify.com/v1/forward', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${key}`
},
body: JSON.stringify({address})
})
.then(response => response.json())
.then(json => console.log(json));
Reverse Geocoding Request
const lat = 37.9358;
const lng = -122.3478;
const key = 'your_geocodify_api_key';
fetch(`https://api.geocodify.com/v1/reverse?lat=${lat}&lng=${lng}`, {
headers: {
'Authorization': `Bearer ${key}`
}
})
.then(response => response.json())
.then(json => console.log(json));
Batch Geocoding Request
const addresses = ['123 Main St, New York, NY', '456 Elm St, Los Angeles, CA'];
const key = 'your_geocodify_api_key';
fetch('https://api.geocodify.com/v1/batch-forward', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${key}`
},
body: JSON.stringify({addresses})
})
.then(response => response.json())
.then(json => console.log(json));
Autocomplete Request
const query = 'Central Park';
const key = 'your_geocodify_api_key';
fetch(`https://api.geocodify.com/v1/autocomplete?q=${query}`, {
headers: {
'Authorization': `Bearer ${key}`
}
})
.then(response => response.json())
.then(json => console.log(json));
Conclusion
The Geocodify API provides a simple and convenient way of accessing geocoding services. The API documentation is well-documented and provides many endpoints for performing geocoding operations, including forward and reverse geocoding, batch geocoding, and autocomplete. By using the API, developers can quickly integrate geocoding capabilities into their own applications, and perform simple, customizable searches for geolocation data.