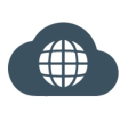
Geolake
GeocodingEasy to use geocoding API with best-in-class accuracy. Provides both API based geocoding as well as via CSV batch upload. Can return ZIP+4 values for US addresses, geocode IP addresses and phone numbers based on the data that is available. Data returned may include the top level domain for a country, currency and time zone for the location.
📚 Documentation & Examples
Everything you need to integrate with Geolake
🚀 Quick Start Examples
// Geolake API Example
const response = await fetch('https://geolake.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring Geolake Public APIs
Geolake is a platform that provides a variety of APIs related to geospatial data. Whether you're building a navigation app or analyzing demographic trends, Geolake's APIs can provide useful data. In this article, we'll explore some of the key Geolake APIs and provide examples of how to use them.
Getting Started
To get started with Geolake APIs, first, you'll need to create an account and obtain an API key. Once you have the API key, you can begin making requests to the APIs.
To make API requests, you can use any programming language that supports HTTP requests. In this article, we'll provide example code written in JavaScript.
Geocoding API
Geocoding API allows you to convert addresses into coordinates (latitude and longitude) and vice versa. Here's an example of how to use Geolake's Geocoding API using JavaScript:
// Example of using Geocoding API
const apiKey = '<YOUR_API_KEY>';
const address = '1600 Amphitheatre Parkway, Mountain View, CA 94043, USA';
const url = `https://api.geolake.com/geocode?address=${address}&apikey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
const location = data.locations[0];
console.log(location.latitude, location.longitude);
})
.catch(error => console.error(error));
In this example, we're using the fetch()
method to make a GET request to the Geocoding API endpoint. We're passing the address to be geocoded as a query parameter and the API key as an authentication parameter. The response from the API is in JSON format, which we're parsing to extract the latitude and longitude.
Routing API
Routing API enables you to calculate the optimal route between two or more points based on various parameters such as distance, time, and traffic. Here's an example of how to use Geolake's Routing API using JavaScript:
// Example of using Routing API
const apiKey = '<YOUR_API_KEY>';
const start = '51.5072,-0.1276'; // London
const end = '48.8566,2.3522'; // Paris
const url = `https://api.geolake.com/route?start=${start}&end=${end}&apikey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
const distance = data.routes[0].distance;
const duration = data.routes[0].duration;
console.log(`Distance: ${distance}, Duration: ${duration}`);
})
.catch(error => console.error(error));
In this example, we're using the fetch()
method to make a GET request to the Routing API endpoint. We're passing the start and end locations as query parameters and the API key as an authentication parameter. The response from the API is in JSON format, which we're parsing to extract the distance and duration of the route.
Demographics API
Demographics API provides detailed demographic data for a given area, such as population, age, education levels, and income. Here's an example of how to use Geolake's Demographics API using JavaScript:
// Example of using Demographics API
const apiKey = '<YOUR_API_KEY>';
const location = '51.5072,-0.1276'; // London
const url = `https://api.geolake.com/demographics?location=${location}&apikey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
const population = data.data.Population;
const income = data.data.Households.Income;
console.log(`Population: ${population}, Income: ${income}`);
})
.catch(error => console.error(error));
In this example, we're using the fetch()
method to make a GET request to the Demographics API endpoint. We're passing the location as a query parameter and the API key as an authentication parameter. The response from the API is in JSON format, which we're parsing to extract the population and income data.
Conclusion
Geolake's APIs provide extensive geospatial data that can be leveraged to create various location-based services. In this article, we explored some of the key APIs, and provided examples of how to use them using JavaScript. With these APIs, developers can create applications that are not only location-aware but also user-centric and dynamic.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes