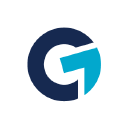
π Documentation & Examples
Everything you need to integrate with GetOTP
π Quick Start Examples
// GetOTP API Example
const response = await fetch('https://otp.dev/en/docs/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
The OTP API provides a streamlined solution for implementing one-time password (OTP) flows in applications, enabling developers to enhance security effortlessly. With support for various authentication methods, this API ensures that users can verify their identities quickly and securely during transactions or sign-ins. By integrating this service, you can reduce vulnerabilities associated with password theft and provide a better user experience that prioritizes safety.
Using the OTP API comes with numerous benefits that can elevate your application's authentication process. Key advantages include the rapid setup of OTP flows, improved security through time-limited codes, flexibility in integration across multiple platforms, compatibility with various communication channels like SMS and email, and comprehensive documentation to guide developers through implementation.
- Rapid Setup: Get started quickly with minimal configuration.
- Enhanced Security: Protect user accounts with time-sensitive, one-time codes.
- Flexible Integration: Easily implement across web and mobile applications.
- Multiple Channels: Send OTPs via SMS, email, or other messaging services.
- Extensive Documentation: Access rich resources for seamless implementation.
Hereβs a simple JavaScript code example for calling the OTP API:
const fetch = require('node-fetch');
async function requestOTP(phoneNumber) {
const response = await fetch('https://otp.dev/api/request', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ phone: phoneNumber }),
});
const data = await response.json();
if (response.ok) {
console.log('OTP sent successfully:', data);
} else {
console.error('Error sending OTP:', data);
}
}
// Usage example
requestOTP('1234567890');
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes