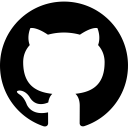
GitHub
DevelopmentThe GitHub API offers a powerful and efficient way to access and manipulate the vast ecosystem of GitHub repositories, user data, and code integrations programmatically. By leveraging this API, developers can automate workflows, interact with GitHub projects, and build applications that seamlessly integrate with the GitHub platform. With detailed endpoints available for repositories, pull requests, issues, and more, users can tailor their interactions to fit the unique needs of their projects. For comprehensive guidance, the official GitHub REST API documentation provides extensive resources, making it easier than ever to get started.
Utilizing the GitHub API comes with numerous benefits for developers and organizations alike. Key advantages include:
- Enhanced Automation: Streamline tasks such as issue tracking and deployment with automated processes.
- Data Insights: Access real-time data and statistics on repository activity, contributions, and user interactions.
- Custom Integrations: Build tailored applications that connect with GitHub to enhance productivity and collaboration.
- User Management: Manage user information and permissions programmatically, simplifying team workflows.
- Open Source Contributions: Easily discover and contribute to open source projects through accessible code repositories.
Here’s a simple JavaScript example demonstrating how to call the GitHub API to fetch user information:
fetch('https://api.github.com/users/octocat')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching user data:', error));