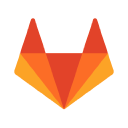
Gitlab
DevelopmentAccess GitLab Functions using REST APIs
GitLab is a well-known web-based Git repository manager, famous for its great features to manage the entire software development lifecycle. GitLab offers a very wide range of APIs to perform any required operation, including manage projects, merge requests, groups, users, issues, jobs, and pipelines. In this blog, we'll go through a few examples of GitLab REST APIs that can be used through JavaScript to access these functions.
To leverage GitLab APIs with JavaScript, you need to follow certain steps, including setting up the user's access token to authenticate, performing the API endpoint call, and parsing the response. Now, let's start with some GitLab API examples in JavaScript.
Example 1 - Get a list of projects
To get a list of GitLab projects using REST APIs, use the following example code in JavaScript:
const url = "https://gitlab.example.com/api/v4/projects";
const accessToken = "your-access-token";
const headers = {
"PRIVATE-TOKEN": accessToken,
};
fetch(url, { headers })
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
In this code, we are setting the url
to the GitLab projects endpoint URL, the accessToken
variable is set as your GitLab access token, and finally, we make an HTTP GET
request to fetch project data, which we console log.
Example 2 - Create an issue on a project
To create an issue on a GitLab project using REST APIs, use the following JavaScript example code:
const url = "https://gitlab.example.com/api/v4/projects/1/issues";
const accessToken = "your-access-token";
const headers = {
"Content-Type": "application/json",
"PRIVATE-TOKEN": accessToken,
};
const issueData = {
title: "Issue title",
description: "Issue description",
assignee_ids: [1],
};
fetch(url, {
method: "POST",
headers,
body: JSON.stringify(issueData),
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
Here, we set the url
to the issue endpoint URL, and the accessToken
variable is set as your GitLab access token. Afterwards, we define the headers
using the Content-Type
and PRIVATE-TOKEN
elements, and then we specify the issueData
object to define the issue attributes. Then, we make an HTTP POST
request with the issue data in JSON format, which we console log.
Conclusion
In this blog, we have covered two examples of GitLab REST APIs using JavaScript for listing projects and creating issues. GitLab provides a vast range of APIs for all the necessary operations. We hope this guide has provided a basic understanding and necessary knowledge for further GitLab API interactions.