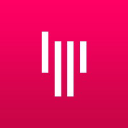
Gitter
DevelopmentThe "Chat for Developers" API is a powerful tool that enables developers to integrate real-time chat functionality into their applications seamlessly. This API is designed to facilitate communication and collaboration within developer-focused environments, making it an essential resource for teams and communities that rely on quick, efficient messaging. With comprehensive documentation available at Gitter Developer Documentation, developers can effortlessly implement chat features, customize their user experience, and foster engagement among their users. The API supports various functionalities, such as creating chat rooms, managing messages, and integrating third-party services, providing developers with the flexibility to build tailored solutions.
Utilizing the "Chat for Developers" API comes with numerous advantages that enhance the development experience. Key benefits include:
- Streamlined integration for real-time communication into existing applications.
- Enhanced team collaboration with the ability to create and manage chat rooms.
- Full control over message management, including retrieval and deletion.
- Customizable user experience with branding and theme options.
- Comprehensive documentation and support resources to ease the implementation process.
Here’s a simple JavaScript example of how to use the API to send a message to a chat room:
const axios = require('axios');
const sendMessage = async (roomId, message) => {
try {
const response = await axios.post(`https://api.gitter.im/v1/rooms/${roomId}/chatMessages`, {
text: message
}, {
headers: {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
});
console.log('Message sent:', response.data);
} catch (error) {
console.error('Error sending message:', error);
}
};
// Example usage:
const roomId = 'YOUR_ROOM_ID';
const message = 'Hello, Gitter!';
sendMessage(roomId, message);