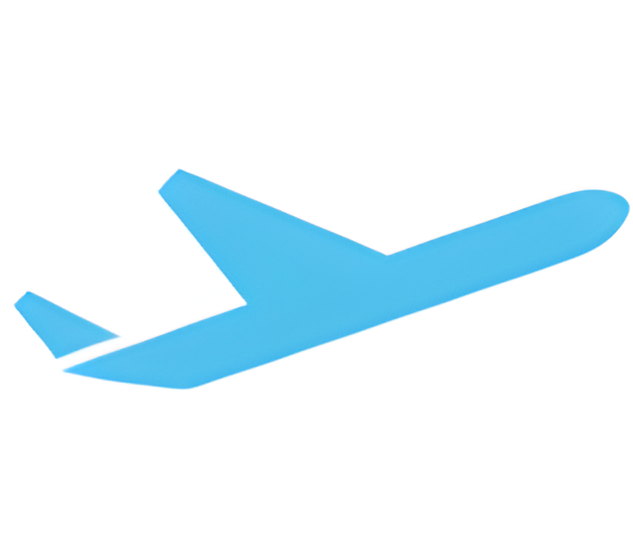
Goflightlabs
TransportationGoFlightLabs is a web-based platform that provides a simple and efficient API for accessing global aviation data in real-time and historical flights. With access to an extensive dataset of airline routes and other aviation-related information, customers can easily tap into the latest data to enhance their flight experience. The platform's user-friendly interface allows users to easily integrate the API into their applications, simplifying the process of data retrieval and analysis. Whether you are an aviation enthusiast, researcher, or business owner, GoFlightLabs offers the perfect solution for accessing up-to-date aviation data.
📚 Documentation & Examples
Everything you need to integrate with Goflightlabs
🚀 Quick Start Examples
// Goflightlabs API Example
const response = await fetch('https://app.goflightlabs.com/dashboard', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using Public APIs of GoFlightLabs
GoFlightLabs offers a Public API that allows users to access their dashboard data programmatically. This API can be used by developers to automate their workflows, retrieve information, integrate it into their applications, and do much more. Furthermore, the API is straightforward and easy to use with any programming language.
Authentication
Before you start using the API, you need to register for an account on the GoFlightLabs website. Once you have created an account, you will obtain an API token that you can use to make requests to the API. To authenticate a request, you need to include your token in the Authorization
header:
Authorization: Bearer <your_token_here>
API Endpoints
These are the available API endpoints that you can use to access the GoFlightLabs data:
- Get User Details: Returns information about the authenticated user.
GET https://app.goflightlabs.com/api/user
Example JS code:
const axios = require('axios').default;
const baseURL = "https://app.goflightlabs.com/api/"
const token = "<your_token_here>";
const options = {
"headers": {
"Authorization": `Bearer ${token}`
}
}
// Make the request
axios.get(baseURL + 'user', options)
.then(response => console.log(response.data))
.catch(error => console.log(error));
- Get Dashboard Details: Returns all the dashboard details of the user.
GET https://app.goflightlabs.com/api/dashboard
Example JS code:
const axios = require('axios').default;
const baseURL = "https://app.goflightlabs.com/api/"
const token = "<your_token_here>";
const options = {
"headers": {
"Authorization": `Bearer ${token}`
}
}
// Make the request
axios.get(baseURL + 'dashboard', options)
.then(response => console.log(response.data))
.catch(error => console.log(error));
- Get Dashboard Details by ID: Returns details for a specific dashboard ID.
GET https://app.goflightlabs.com/api/dashboard/:id
Example JS code:
const axios = require('axios').default;
const baseURL = "https://app.goflightlabs.com/api/"
const token = "<your_token_here>";
const dashboardID = "<dashboard_id_here>";
const options = {
"headers": {
"Authorization": `Bearer ${token}`
}
}
// Make the request
axios.get(baseURL + `dashboard/${dashboardID}`, options)
.then(response => console.log(response.data))
.catch(error => console.log(error));
- Create a New Dashboard: Creates a new dashboard and returns its details.
POST https://app.goflightlabs.com/api/dashboard
Example JS code:
const axios = require('axios').default;
const baseURL = "https://app.goflightlabs.com/api/"
const token = "<your_token_here>";
const dashboardData = {
"name": "New Dashboard",
"description": "This is a new dashboard created via the API",
"data": {}
}
const options = {
"headers": {
"Authorization": `Bearer ${token}`
}
}
// Make the request
axios.post(baseURL + 'dashboard', dashboardData, options)
.then(response => console.log(response.data))
.catch(error => console.log(error));
- Update an Existing Dashboard: Updates the details of an existing dashboard and returns its updated details.
PUT https://app.goflightlabs.com/api/dashboard/:id
Example JS code:
const axios = require('axios').default;
const baseURL = "https://app.goflightlabs.com/api/"
const token = "<your_token_here>";
const dashboardID = "<dashboard_id_here>";
const updatedData = {
"name": "Updated Dashboard",
"description": "This dashboard has been updated via the API",
"data": {}
}
const options = {
"headers": {
"Authorization": `Bearer ${token}`
}
}
// Make the request
axios.put(baseURL + `dashboard/${dashboardID}`, updatedData, options)
.then(response => console.log(response.data))
.catch(error => console.log(error));
- Delete an Existing Dashboard: Deletes an existing dashboard.
DELETE https://app.goflightlabs.com/api/dashboard/:id
Example JS code:
const axios = require('axios').default;
const baseURL = "https://app.goflightlabs.com/api/"
const token = "<your_token_here>";
const dashboardID = "<dashboard_id_here>";
const options = {
"headers": {
"Authorization": `Bearer ${token}`
}
}
// Make the request
axios.delete(baseURL + `dashboard/${dashboardID}`, options)
.then(response => console.log(response.data))
.catch(error => console.log(error));
Conclusion
This blog post has covered several API endpoints available from the GoFlightLabs Public API. These endpoints allow users to access dashboard data programmatically. Moreover, this post includes examples of JS code that could be run to achieve the same results. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes