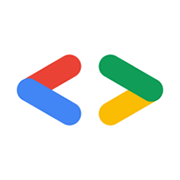
Google Books
BooksExploring the Google Books API
The Google Books API is a powerful tool for accessing data about books from the Google Books database. In this blog post, we'll explore some of the key features of the API and provide example code in JavaScript to get you started.
Getting started
To get started with the Google Books API, you first need to create a project in the Google Cloud Console. Once you have created a project, you can enable the Google Books API and obtain an API key.
With your API key in hand, you can start making requests to the Google Books API. All requests start with the base URL https://www.googleapis.com/books/v1
and are followed by the endpoint for the specific resource you want to access.
For example, to get information about a specific book by its ISBN, you would make a GET request to the following URL:
https://www.googleapis.com/books/v1/volumes?q=isbn:9781451648546&key=YOUR_API_KEY
Performing a search
To perform a search for books, you can use the volumes
endpoint and provide a query parameter. For example, the following code performs a search for books containing the word "javascript":
const search = async () => {
const response = await fetch(`https://www.googleapis.com/books/v1/volumes?q=javascript&key=YOUR_API_KEY`);
const data = await response.json();
console.log(data);
}
This code uses the fetch
API to make a GET request to the Google Books API and retrieve the results. The results are returned as a JSON object, which we can log to the console for further processing.
Accessing book details
Once you have retrieved a list of books, you can access the details for each book by its ID. For example, the following code retrieves the details for a specific book with the ID EMMWDwAAQBAJ
:
const getBookDetails = async () => {
const response = await fetch(`https://www.googleapis.com/books/v1/volumes/EMMWDwAAQBAJ?key=YOUR_API_KEY`);
const data = await response.json();
console.log(data);
}
This code uses the volumes
endpoint with the book's ID to retrieve the details for that specific book. Again, the results are returned as a JSON object which we can log to the console.
Conclusion
The Google Books API is a powerful tool for accessing data about books. In this post, we've explored some of the key features of the API and provided example code in JavaScript for performing searches and accessing book details.
If you're interested in learning more about the Google Books API, be sure to check out the official documentation. Happy coding!