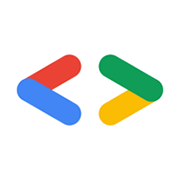
Google Drive
Cloud Storage & File SharingUsing the Google Drive API with JavaScript
If you want to integrate your web application with Google Drive, you'll need to use the Google Drive API. This API allows you to create, read, update, and delete files stored in a user's Google Drive account.
In this article, we'll cover some basic examples of how to use the Google Drive API with JavaScript.
Prerequisites
Before you get started, you'll first need to create a project in the Google Cloud Console and enable the Google Drive API for that project. After that, you'll need to create credentials for your project and obtain an access token. You can find detailed instructions for doing this in the Google Drive API documentation.
Once you have your access token, you're ready to start using the Google Drive API in your JavaScript code.
Listing Files
To list the files in a user's Google Drive account, you can use the gapi.client.drive.files.list
method. Here's an example of how to do this:
function listFiles() {
gapi.client.drive.files.list({
'pageSize': 10,
'fields': "nextPageToken, files(id, name)"
}).then(function(response) {
var files = response.result.files;
console.log("Files:");
if (files && files.length > 0) {
for (var i = 0; i < files.length; i++) {
var file = files[i];
console.log(file.name + ' (' + file.id + ')');
}
} else {
console.log('No files found.');
}
});
}
This function uses the pageSize
parameter to limit the number of results returned, and the fields
parameter to specify which fields should be included in the response. In this case, we're only interested in the file IDs and names.
Uploading Files
To upload a file to a user's Google Drive account, you can use the gapi.client.drive.files.create
method. Here's an example of how to do this:
function uploadFile(fileContent, fileName) {
var fileMetadata = {
'name': fileName
};
var media = {
mimeType: 'text/plain',
body: fileContent
};
gapi.client.drive.files.create({
resource: fileMetadata,
media: media,
fields: 'id'
}).then(function(response) {
console.log('File ID: ' + response.result.id);
});
}
This function takes two parameters: the content of the file (in this case, plain text) and the file name. It then creates a file metadata object and a media object for the file and calls the create
method with those objects.
Downloading Files
To download a file from a user's Google Drive account, you can use the gapi.client.drive.files.get
method. Here's an example of how to do this:
function downloadFile(fileId) {
gapi.client.drive.files.get({
fileId: fileId,
alt: 'media'
}).then(function(response) {
console.log(response.body);
});
}
This function takes a single parameter: the ID of the file to download. It then calls the get
method with that ID and the alt
parameter set to media
, which specifies that we want to download the file's contents rather than just the metadata.
Conclusion
With these examples, you should now have a basic understanding of how to use the Google Drive API with JavaScript. Be sure to check out the official documentation for more information and additional API methods.