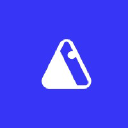
GraphQL Jobs
JobsThe "Jobs with GraphQL" API offers a powerful way to access job listings in your applications. Built on GraphQL, this API allows developers to retrieve job data efficiently, providing a flexible and efficient alternative to traditional REST APIs. Users can filter and search job offerings based on various criteria, making it a perfect fit for job boards, recruitment platforms, and anyone looking to integrate job-related data into their services. With its well-structured documentation, developers can quickly understand the use cases, query capabilities, and responses, enabling them to implement job searches seamlessly.
Using the "Jobs with GraphQL" API comes with several benefits, including:
- Flexibility in querying various fields without requiring multiple endpoints.
- Reduced data over-fetching and under-fetching, leading to optimized performance.
- A strong and active community for support and improvements.
- Real-time job listings that are updated frequently for accuracy.
- Easy integration into existing applications with clear and concise schema definitions.
Here’s a simple JavaScript code example to call the "Jobs with GraphQL" API:
const fetch = require('node-fetch');
const query = `
{
jobs {
id
title
location
company {
name
website
}
}
}
`;
fetch('https://graphql.jobs/api', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ query }),
})
.then(response => response.json())
.then(data => {
console.log('Jobs:', data.data.jobs);
})
.catch(error => console.error('Error fetching jobs:', error));