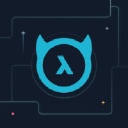
Hasura
DevelopmentThe GraphQL and REST API Engine with built-in Authorization offers a powerful solution for developers looking to streamline their backend operations. This robust engine allows users to create, modify, and manage APIs effortlessly while ensuring secure access through integrated authorization features. By offering both GraphQL and REST interfaces, it caters to diverse development needs, enabling teams to leverage the flexibility of GraphQL’s query language or the simplicity of traditional REST endpoints. With comprehensive documentation available at Hasura's official site, developers can quickly get started and tap into all the functionalities the API engine has to offer.
Utilizing this API Engine brings numerous advantages, making it an ideal choice for modern application development. Benefits include a significant reduction in development time, enhanced security through built-in authentication mechanisms, automatic handling of real-time data through subscriptions, seamless integration with existing PostgreSQL databases, and an intuitive interface that simplifies API exploration and testing. Below is a JavaScript code snippet demonstrating how to call the API effectively:
const fetch = require('node-fetch');
const query = `
query {
users {
id
name
email
}
}
`;
fetch('https://your-api-endpoint.com/v1/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
},
body: JSON.stringify({ query })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));