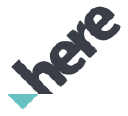
HERE Maps
GeocodingHERE Maps API: A Comprehensive Guide
HERE Maps API is a popular mapping and geolocation API designed to help developers build innovative and feature-rich applications. This public API offers a wide range of features that help developers to create customized maps, geocode addresses, and access real-time traffic data. In this blog post, we explore some of the key features of the HERE Maps API and provide a comprehensive guide on how to use it.
Getting Started with HERE Maps API
Before we dive into the detailed features, let's start with setting up the HERE Maps API. Here's how you can get started:
- Sign up for an account on the HERE Developer Website. You will need your API key in order to use the API.
- Choose the type of API you want to use, depending on your application requirements. You can choose from a wide range of APIs such as maps, routing, geocoding, traffic, and more.
- To use the HERE Maps API in JavaScript, you need to include the HERE Maps JavaScript API Library in your application. You can use the following code to include the library in your HTML file:
<script src="https://js.api.here.com/v3/3.1/mapsjs-core.js"
type="text/javascript" charset="utf-8"></script>
<script src="https://js.api.here.com/v3/3.1/mapsjs-service.js"
type="text/javascript" charset="utf-8"></script>
<script src="https://js.api.here.com/v3/3.1/mapsjs-ui.js"
type="text/javascript" charset="utf-8"></script>
Displaying a Map
One of the most basic features of the HERE Maps API is displaying a map. You can use the following code to display a map on your web page:
// Specify the container for the map.
var container = document.getElementById('map');
// Specify the center and zoom level for the map.
var center = {lat: 52.520008, lng: 13.404954};
var zoom = 14;
// Create a new instance of the map.
var map = new H.Map(container, maptypes.normal.map, {
center: center,
zoom: zoom
});
Markers and Geocoding
You can also add markers and geocode addresses using the HERE Maps API. Here's how you can do that:
// Geocode an address.
var geocoder = platform.getGeocodingService();
geocoder.geocode(
{ searchText: '200 S Mathilda Ave, Sunnyvale, CA 94086' },
function(result) {
// Add a marker to the location of the geocoded address.
var marker = new H.map.Marker(result.items[0].position);
map.addObject(marker);
}, function(error) {
console.log(error);
}
);
Routing
Another useful feature of the HERE Maps API is routing. You can use the API to calculate and display the fastest route between two points on a map. Here's how you can do that:
// Calculate the fastest route.
var router = platform.getRoutingService();
var routeRequestParams = {
mode: 'fastest;car',
representation: 'display',
routeAttributes: 'summary'
};
var onResult = function(result) {
// Plot the route on the map.
var route = result.response.route[0];
route.sections.forEach(function(section) {
var linestring = H.geo.LineString.fromFlexiblePolyline(section.polyline);
var polyline = new H.map.Polyline(linestring, {
style: { strokeColor: 'blue', lineWidth: 3 }
});
map.addObject(polyline);
});
};
router.calculateRoute(routeRequestParams, onResult, function(error) {
console.log(error);
});
Real-Time Traffic
You can also use the HERE Maps API to access real-time traffic data. Here's how you can do that:
// Load traffic tiles.
var trafficProvider = new H.service.TrafficService({
apikey: '{YOUR_API_KEY}'
});
var trafficLayer = trafficProvider.createTileLayer({
tileType: 'flowtile',
min: 5,
max: 50
});
map.addLayer(trafficLayer);
Conclusion
The HERE Maps API is a powerful and user-friendly API designed to take care of all your geolocation, routing, and mapping needs. With the extensive documentation and intuitive examples, it is easy for developers to get started and build exceptional mapping applications. We hope this guide has been a helpful introduction to the HERE Maps API and its vast capabilities.