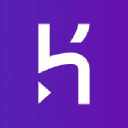
Heroku
DevelopmentThe Heroku REST API empowers developers to interact with the Heroku platform programmatically, allowing for seamless app creation, provisioning of add-ons, and execution of various tasks essential for application management. With its comprehensive set of endpoints, the API facilitates efficient workflows, enabling developers to automate their processes and integrate Heroku functionalities into their applications. This not only accelerates development cycles but also enhances operational efficiency, making it a valuable tool for businesses aiming to optimize their cloud application deployment and management strategies.
Utilizing the Heroku REST API brings numerous advantages:
- Simplifies the app deployment process through automation.
- Facilitates the seamless integration of third-party add-ons to enhance app functionality.
- Allows for easy monitoring and scaling of applications, ensuring optimal performance.
- Provides comprehensive management capabilities across multiple Heroku applications.
- Offers robust documentation and community support for quick troubleshooting.
Here’s a JavaScript code example demonstrating how to call the Heroku REST API:
const axios = require('axios');
const herokuApiKey = 'YOUR_HEROKU_API_KEY';
const appName = 'YOUR_APP_NAME';
const apiUrl = `https://api.heroku.com/apps/${appName}`;
axios.get(apiUrl, {
headers: {
Authorization: `Bearer ${herokuApiKey}`,
Accept: 'application/vnd.heroku+json; version=3',
}
})
.then(response => {
console.log('App Details:', response.data);
})
.catch(error => {
console.error('Error fetching app details:', error);
});