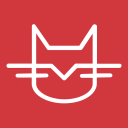
HTTPCat
AnimalsPlaying with API Docs of HTTP.Cat
HTTP.Cat is a fun API website that provides HTTP status codes as cute cat images. Yes, this is a real thing! You can use this API in your projects to add some humor or to make error handling more fun. In this article, we will explore HTTP.Cat API documentation and learn how to make API requests in JavaScript.
API Docs
The HTTP.Cat documentation page is very straightforward. You can find all available HTTP status codes along with their corresponding cat images. The API endpoint is https://http.cat/{status_code}.jpg. For example, to get the cat image for the 404 error, you can use the URL https://http.cat/404.jpg.
Example Code
To make an API request to HTTP.Cat in JavaScript, you can use the built-in fetch
function. Here is an example code snippet that demonstrates how to request the cat image for the 404 status code:
const statusCode = 404;
const url = `https://http.cat/${statusCode}.jpg`;
// Request the cat image from the API endpoint
fetch(url)
.then((response) => {
if (response.ok) {
// If the response was successful, display the cat image
const img = document.createElement('img');
img.src = url;
document.body.appendChild(img);
} else {
// If there was an error with the request, log the error message
console.log(`Error: ${response.statusText}`);
}
})
.catch((error) => {
console.log(`Error: ${error}`);
});
This code creates an img
element and sets its src
attribute to the API endpoint URL. Then it makes a fetch
request to that URL and handles the response. If the response is successful, it displays the cat image. If there is an error with the request, it logs the error message to the console.
Conclusion
HTTP.Cat API is a fun way to add some humor to your projects. Its documentation is straightforward and easy to understand. In this article, we have learned how to make API requests to HTTP.Cat in JavaScript using the fetch
function. Have fun playing with this API!