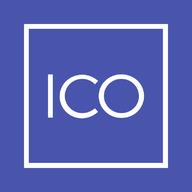
ICObench
CryptocurrencyAccessing ICOBench API with JavaScript
ICOBench is a comprehensive platform that provides information about initial coin offerings (ICOs), cryptocurrency exchanges, and blockchain startups. The platform has a public API that enables users to access ICOBench's database.
In this article, we will explore how to access ICOBench's API using JavaScript. We will cover authentication, endpoint URLs, and sample code for accessing different endpoints.
Authentication
ICOBench's API requires a secret key for authentication. To obtain a secret key, you need to sign up on ICOBench's website and create an API key. ICOBench's API uses a basic authentication scheme that expects the secret key to be sent as a header. The following code snippet shows how to set up the authentication header:
const API_KEY = '<YOUR_API_KEY>';
const headers = {
'Authorization': 'Basic ' + btoa(API_KEY + ':')
};
Endpoint URLs
ICOBench's API supports different endpoints for ICOs, exchanges, and startups. Each endpoint has a unique URL that starts with https://icobench.com/api/v1/
. Here are some examples of endpoint URLs:
https://icobench.com/api/v1/icos
for retrieving information about ICOshttps://icobench.com/api/v1/exchanges
for retrieving information about cryptocurrency exchangeshttps://icobench.com/api/v1/startups
for retrieving information about blockchain startups
Each endpoint URL may have additional parameters that allow you to filter the results. For example, you can filter ICOs by their status, category, or country. You can find the list of available parameters in ICOBench's API documentation.
Sample Code
The following code snippets show how to access ICOBench's API using JavaScript. We will retrieve information about ICOs, exchanges, and startups.
Retrieving ICOs
To retrieve information about ICOs, we will send a GET request to the https://icobench.com/api/v1/icos
endpoint. The following code snippet shows how to retrieve the top 10 ICOs by rating:
const URL = 'https://icobench.com/api/v1/icos?rating=top5';
const headers = {
'Authorization': 'Basic ' + btoa(API_KEY + ':')
};
fetch(URL, {headers})
.then(response => response.json())
.then(data => {
// Process the ICO data
console.log(data);
});
Retrieving Exchanges
To retrieve information about cryptocurrency exchanges, we will send a GET request to the https://icobench.com/api/v1/exchanges
endpoint. The following code snippet shows how to retrieve the top 10 exchanges by volume:
const URL = 'https://icobench.com/api/v1/exchanges?volume=top10';
const headers = {
'Authorization': 'Basic ' + btoa(API_KEY + ':')
};
fetch(URL, {headers})
.then(response => response.json())
.then(data => {
// Process the exchange data
console.log(data);
});
Retrieving Startups
To retrieve information about blockchain startups, we will send a GET request to the https://icobench.com/api/v1/startups
endpoint. The following code snippet shows how to retrieve the top 10 startups by score:
const URL = 'https://icobench.com/api/v1/startups?score=top10';
const headers = {
'Authorization': 'Basic ' + btoa(API_KEY + ':')
};
fetch(URL, {headers})
.then(response => response.json())
.then(data => {
// Process the startup data
console.log(data);
});
Conclusion
ICOBench's API is a powerful tool for developers who need to access information about ICOs, exchanges, and startups. In this article, we have covered authentication, endpoint URLs, and sample code for accessing different endpoints. We hope this article will help you get started with ICOBench's API and build amazing applications.