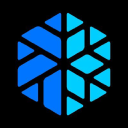
icy.tools
CryptocurrencyThe GraphQL-based NFT API offers a powerful and flexible solution for developers and businesses looking to integrate Non-Fungible Token (NFT) capabilities into their applications. By leveraging the GraphQL interface, users can query specific data points and handle complex interactions with NFTs more efficiently than traditional REST APIs. This advanced API streamlines access to NFT data, including metadata, ownership, and transaction history, making it an essential tool for building innovative blockchain applications. For developers, the API provides comprehensive documentation available here to ensure a seamless integration process.
Utilizing this API comes with numerous advantages that enhance the development experience. Some key benefits include:
- Efficient Data Retrieval: Fetch only the data you need, reducing bandwidth and improving performance.
- Real-Time Data Access: Stay up-to-date with the latest NFT trends and transactions.
- User-Friendly Queries: Simplify complex data queries with the power of GraphQL's intuitive syntax.
- Enhanced Flexibility: Easily adapt your API calls based on changing application requirements.
- Strong Community Support: Engage with an active community and access resources for troubleshooting and enhancements.
Here’s a simple JavaScript code example for calling the GraphQL NFT API:
const fetch = require('node-fetch');
const query = `
query {
nfts(first: 5) {
id
name
owner {
id
}
}
}
`;
fetch('https://api.icy.tools/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ query }),
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching NFTs:', error));