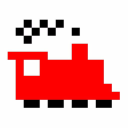
Indian Railways
TransportationExploring the eRail API using JavaScript
The eRail API is a public API that provides users with real-time information on Indian Railways. This API allows users to access data on train schedules, fares, and routes, as well as information on the availability of seats and train coaches. With this API, users can retrieve information on over 7,500 trains and more than 22,000 stations across India.
In this tutorial, we will explore the eRail API using JavaScript code and see how we can use the API to retrieve data in a JSON format. We will cover the following topics:
- Getting Started
- Retrieving Train Information
- Retrieving Station Information
- Retrieving Train Schedule Information
- Retrieving Train Fares Information
Getting Started
Before we can start using the eRail API, we need to obtain an API key. To get an API key, simply sign up for an account on http://api.erail.in/auth/register and follow the instructions.
Once you have your API key, you can start using the eRail API to retrieve train, station, schedule, and fare information in JSON format.
To retrieve information from the eRail API using JavaScript, we will use the Fetch API
. The Fetch API
is a simplified interface for making HTTP requests and handling responses. You can learn more about the Fetch API
in the MDN Web Docs.
To get started, create a new HTML file and add the following code to it:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>eRail API Example</title>
</head>
<body>
<h1>eRail API Example</h1>
<script>
const apiUrl = 'http://api.erail.in/';
const apiKey = 'YourAPIKeyHere';
</script>
</body>
</html>
In the above code, replace apiKey
with your API key.
Retrieving Train Information
To retrieve information about a specific train, we can use the Live Trains
API endpoint. The Live Trains
API endpoint returns a list of trains that are running on a specific date between two specified stations. The endpoint takes the following parameters:
key
: The eRail API key.stnfrom
: The station code of the departure station.stnto
: The station code of the destination station.date
: The date of journey indd-mm-yyyy
format.sort
: The field to sort the results by. This can betrainno
,src
,dest
,depttime
, orarrtime
.order
: The order to sort the results in. This can beasc
for ascending ordesc
for descending.
To retrieve information about a specific train, add the following code to your HTML file:
<script>
const trainNo = '12345';
const date = '01-01-2022';
fetch(`${apiUrl}live-train-status/train/${trainNo}/date/${date}/apikey/${apiKey}/`)
.then(response => response.json())
.then(data => console.log(data));
</script>
In the above code, replace trainNo
and date
with the desired values.
Retrieving Station Information
To retrieve information about a specific station, we can use the Stations
API endpoint. The Stations
API endpoint returns a list of stations based on the search query. The endpoint takes the following parameters:
key
: The eRail API key.stnName
: The name of the station to search for.
To retrieve information about a specific station, add the following code to your HTML file:
<script>
const stationName = 'delhi';
fetch(`${apiUrl}stations/?names=${stationName}&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
</script>
In the above code, replace stationName
with the desired station name.
Retrieving Train Schedule Information
To retrieve the schedule of a specific train, we can use the Train Schedule
API endpoint. The Train Schedule
API endpoint returns the schedule of a specific train for a specified date. The endpoint takes the following parameters:
key
: The eRail API key.trainid
: The train number.date
: The date of journey indd-mm-yyyy
format.
To retrieve the schedule of a specific train, add the following code to your HTML file:
<script>
const trainNo = '12345';
const date = '01-01-2022';
fetch(`${apiUrl}train-schedule/train/${trainNo}/date/${date}/apikey/${apiKey}/`)
.then(response => response.json())
.then(data => console.log(data));
</script>
In the above code, replace trainNo
and date
with the desired values.
Retrieving Train Fares Information
To retrieve the fares of a specific train, we can use the Fares
API endpoint. The Fares
API endpoint returns the fares of a specific train for a specified date. The endpoint takes the following parameters:
key
: The eRail API key.trainid
: The train number.stnfrom
: The station code of the departure station.stnto
: The station code of the destination station.date
: The date of journey indd-mm-yyyy
format.
To retrieve the fares of a specific train, add the following code to your HTML file:
<script>
const trainNo = '12345';
const stnFrom = 'NDLS';
const stnTo = 'BCT';
const date = '01-01-2022';
fetch(`${apiUrl}fare/${trainNo}/${stnFrom}/${stnTo}/${date}/apikey/${apiKey}/`)
.then(response => response.json())
.then(data => console.log(data));
</script>
In the above code, replace trainNo
, stnFrom
, stnTo
, and date
with the desired values.
Conclusion
In this tutorial, we covered the basics of using the eRail API using JavaScript and the Fetch API
. We explored how we can retrieve train, station, schedule, and fare information using different API endpoints. This is just the tip of the iceberg in terms of what you can do with the eRail API, and we encourage you to explore the API further and build your own applications using the data it provides.