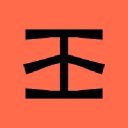
INFURA Ethereum
CryptocurrencyThe Ethereum API offers seamless interaction with the Ethereum mainnet as well as various testnets, enabling developers to build and deploy decentralized applications with ease. By leveraging this robust API, users can access core functionalities of the Ethereum blockchain, including sending transactions, querying smart contracts, and managing wallet operations. With Infura’s infrastructure, developers can ensure high availability and reliability, allowing them to focus on building innovative solutions without the need to maintain their own node.
Utilizing this powerful API comes with numerous benefits, enhancing the development process for blockchain applications. Key advantages include:
- Quick and easy access to both Ethereum mainnet and testnets.
- Reduced infrastructure costs with reliable, scalable solutions.
- Simplified integrations with comprehensive documentation and support.
- Enhanced security features to protect sensitive data during transactions.
- High-performance requests handling thousands of transactions per second.
Here’s a simple JavaScript code example demonstrating how to call the Ethereum API:
const Web3 = require('web3');
const INFURA_URL = 'https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID';
const web3 = new Web3(new Web3.providers.HttpProvider(INFURA_URL));
async function getLatestBlock() {
try {
const latestBlock = await web3.eth.getBlock('latest');
console.log('Latest Block:', latestBlock);
} catch (error) {
console.error('Error fetching the latest block:', error);
}
}
getLatestBlock();
This example illustrates how to connect to the Ethereum mainnet using Infura and fetch the latest block information.