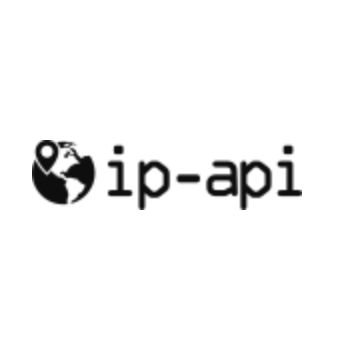
IP Api
GeocodingA Quick Guide to Using the IP-API Public API
Are you looking for a reliable IP location API? Look no further than IP-API! With its simple design and comprehensive documentation, it's a top choice for developers and website owners alike.
In this guide, we'll show you how to get started with IP-API using JavaScript code examples.
How to Use IP-API
To use IP-API, simply make an HTTP GET request to the API endpoint (http://ip-api.com/json/{ip}), where {ip} is the IP address you want to look up. Here's an example:
const ip = "8.8.8.8"
const url = `http://ip-api.com/json/${ip}`
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
This code sets the IP address to "8.8.8.8" and creates the API URL by appending the IP address to the API endpoint. It then uses the fetch
function to make the HTTP GET request and returns the response as JSON data.
The result is a JSON object containing information about the specified IP address. For example:
{
"status": "success",
"country": "United States",
"region": "California",
"city": "Mountain View",
"zip": "94035",
"lat": 37.422,
"lon": -122.084,
"timezone": "America/Los_Angeles"
}
Here, we can see that the IP address 8.8.8.8 is located in Mountain View, California and has a latitude of 37.422 and a longitude of -122.084.
Customizing the API Response
IP-API allows you to customize the API response by passing additional parameters as part of the API URL. Here are a few examples:
Adding Language Information
const ip = "8.8.8.8"
const lang = "en"
const url = `http://ip-api.com/json/${ip}?lang=${lang}`
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
This code sets the language to "en" and adds it to the API URL as a query parameter. The result will now include additional information, such as the country name in English.
Adding Security Information
const ip = "8.8.8.8"
const security = true
const url = `http://ip-api.com/json/${ip}?security=${security}`
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
This code sets the security parameter to "true" and adds it to the API URL as a query parameter. The result will now include additional information about the security of the specified IP address, such as the ISP's name.
Adding Specific Fields
const ip = "8.8.8.8"
const fields = "status,country,regionName"
const url = `http://ip-api.com/json/${ip}?fields=${fields}`
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
This code sets the fields parameter to "status,country,regionName" and adds it to the API URL as a query parameter. The result will now include only the specified fields of information, rather than the full response.
Conclusion
In this guide, we've shown you how to get started with IP-API using JavaScript code examples. With its simple API and comprehensive documentation, IP-API is a great choice for anyone looking to quickly and easily locate and gather information about IP addresses.