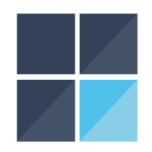
IP Finder
DevelopmentA Beginner's Guide to the ipfinder.io Public API
If you're accessing the internet, there's a good chance you're using an IP address to do so. This unique identifier is assigned to every device that connects to the internet, and it can reveal a lot about your location and browsing history. With the ipfinder.io public API, developers can access information about IP addresses and use it to perform a variety of tasks. In this guide, we'll go over the basic structure of the ipfinder.io API, how to get an API key, and some example code in JavaScript.
Getting Started
Before you can use the ipfinder.io API, you need to sign up for an API key. You can do so by visiting the ipfinder.io website and creating an account. Once you have an account, navigate to the API documentation page to get started.
API Structure
The ipfinder.io API is organized into different endpoints, each of which provides access to different types of information about IP addresses. The basic structure of an API request is as follows:
https://api.ipfinder.io/{endpoint}/{method}?{parameters}&token={API_key}
{endpoint}
refers to the specific endpoint you're accessing (e.g. ipaddress
)
{method}
refers to the specific method you're calling within that endpoint (e.g. getAsn
)
{parameters}
refers to any additional parameters required for that method (e.g. ip=1.2.3.4
)
{API_key}
refers to your personal API key, which is required for all API requests
Example Code
Here's some example code in JavaScript that demonstrates how to use the ipfinder.io API to get information about an IP address:
const baseURL = "https://api.ipfinder.io/";
const endpoint = "ipaddress/";
const method = " getAddressInfo";
const params = "?ip=1.2.3.4";
const apiKey = "&token=your_api_key_here";
const url = `${baseURL}${endpoint}${method}${params}${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
In this code, we first define the baseURL
as the ipfinder.io API URL, then specify the endpoint
, method
, params
, and apiKey
variables. We combine these variables into a single url
variable and use the fetch
function to make a GET request. Finally, we log the response data to the console.
This is just one example of how to use the ipfinder.io API in JavaScript. By exploring the different endpoints and methods provided by the API, you can create even more powerful tools and applications.
Conclusion
The ipfinder.io public API is a valuable resource for developers who need to work with IP addresses. In this short guide, we've covered the basics of how to get started with the API and provided some example code in JavaScript. By exploring the documentation and trying out different requests, you can begin to unlock the full potential of this powerful tool.