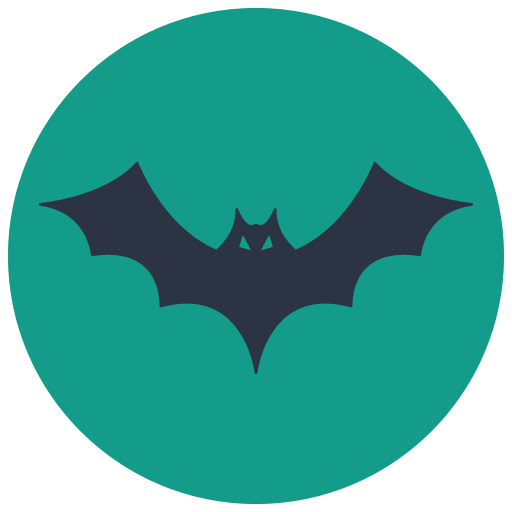
IP Vigilante
GeocodingIntroduction to IP Vigilante API
IP Vigilante is a powerful, public API that helps to secure your web applications and websites from potential cyber threats. It offers various endpoints to lookup a visitors' IP address, validate email addresses, and check if an IP has been recorded as spam or not. IP Vigilante API provides both JSON and XML output formats.
In this blog post, we will discuss the various endpoints provided by the IP Vigilante API along with some JavaScript code examples.
IP Lookup Endpoint
The IP lookup endpoint is used to retrieve information about any IP address. This endpoint returns the country, region, city, time zone, latitude, longitude, and ISP of the IP address.
JavaScript API example
const apiUrl = `https://ipvigilante.com/json/${ipAddress}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
Email Validation Endpoint
The Email Validation endpoint allows you to verify an email address. It returns a boolean status along with a message describing the validation result.
JavaScript API example
const apiUrl = `https://ipvigilante.com/api/email-validate/?email=${emailAddress}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
Spam IP Check Endpoint
The Spam IP Check endpoint checks if an IP address has been marked as spam. A JSON message containing a 'status' value is returned, indicating whether the IP address is spam or not.
JavaScript API example
const apiUrl = `https://ipvigilante.com/api/spam-filter/${ipAddress}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
Conclusion
The IP Vigilante API provides developers with a powerful way to protect their web applications and websites from potential threats. This API is easy to use, and with our JavaScript code examples, you can easily integrate it into your applications. Head over to their website to learn more about their API.