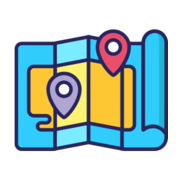
IPGeolocationAPI.com
GeocodingIntroduction to IP Geolocation API
IP Geolocation API offers a simple, yet powerful RESTful API interface to retrieve accurate location data of any IP address. With a fast and reliable service, the IP Geolocation API provides comprehensive geographical information, such as country, city, latitude and longitude, timezone, ISP, domain name, and more. In this blog post, we will explore the different types of API requests and responses that can be performed using IP Geolocation API in JavaScript.
Using IP Geolocation API in JavaScript
Prerequisites
Before we start writing the API code, we need to obtain an API key from https://ipgeolocationapi.com/. The API key will be needed to authenticate the API requests.
Performing IP Geolocation Lookup
To perform an IP geolocation lookup, we need to make an HTTP GET request to the API endpoint with the IP address we want to geolocate. The response will be in JSON format that contains all the geographical information for that IP address.
const apiKey = 'your-api-key';
const ipAddress = '8.8.8.8';
const url = `https://api.ipgeolocationapi.com/geolocate/${ipAddress}?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example code, we are calling the fetch() method with the API endpoint URL and the API key as URL parameters. We then parse the JSON response returned by the server and log it to the console. Note that this request will only return the geolocation data for the IP address specified and not any other details.
Performing Bulk IP Geolocation Lookup
To perform a bulk IP geolocation lookup, we need to make an HTTP POST request to the API with a list of IP addresses we want to geolocate. The response will be in a JSON array format that contains all the geographical information for each IP address.
const apiKey = 'your-api-key';
const url = `https://api.ipgeolocationapi.com/geolocate-many?api_key=${apiKey}`;
const ipList = ['8.8.8.8', '1.1.1.1', '2a00:1450:400e:805::200e'];
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
ip: ipList
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example code, we are calling the fetch() method with the API endpoint URL and the API key as URL parameters. We then specify the HTTP method as POST and include the Content-Type header as "application/json". We also include the list of IP addresses to geolocate in the request body as a JSON object. We then parse the JSON response returned by the server and log it to the console. Note that the bulk lookup request allows up to 1000 IP addresses in a single request.
Conclusion
IP Geolocation API provides a simple and reliable API solution for IP geolocation lookup. In this blog post, we have explored how to perform IP geolocation lookup and bulk IP geolocation lookup requests using JavaScript. With these API requests, we can easily obtain comprehensive geographical information for any IP address of interest.