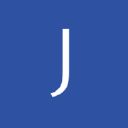
Jikan MyAnimeList Unofficial API
AnimeIntroduction to Jikan API
Jikan is a public API that provides anime and manga data. It was created to provide developers with a simple interface to retrieve useful information about anime and manga.
In this blog, we will explore how to use Jikan API with JavaScript. We will cover different endpoints and their usage with example code.
Getting Started
Before we begin, we need to get an API key from Jikan API website. You can get your API key by visiting https://jikan.moe.
Example Code
Searching for Anime
To search for an anime, we can use the search
endpoint.
async function searchAnime(query) {
const url = `https://api.jikan.moe/v3/search/anime?q=${query}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
searchAnime("naruto")
.then((data) => console.log(data))
.catch((error) => console.log(error));
Fetching Anime Details
To retrieve anime details, we can use the anime
endpoint.
async function getAnimeDetails(id) {
const url = `https://api.jikan.moe/v3/anime/${id}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
getAnimeDetails(20)
.then((data) => console.log(data))
.catch((error) => console.log(error));
Fetching Anime Episodes
To retrieve anime episodes, we can use the episodes
endpoint.
async function getAnimeEpisodes(id, page = 1) {
const url = `https://api.jikan.moe/v3/anime/${id}/episodes/${page}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
getAnimeEpisodes(20, 1)
.then((data) => console.log(data))
.catch((error) => console.log(error));
Searching for Manga
To search for a manga, we can use the search
endpoint.
async function searchManga(query) {
const url = `https://api.jikan.moe/v3/search/manga?q=${query}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
searchManga("one piece")
.then((data) => console.log(data))
.catch((error) => console.log(error));
Fetching Manga Details
To retrieve manga details, we can use the manga
endpoint.
async function getMangaDetails(id) {
const url = `https://api.jikan.moe/v3/manga/${id}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
getMangaDetails(13)
.then((data) => console.log(data))
.catch((error) => console.log(error));
Fetching Manga Chapters
To retrieve manga chapters, we can use the chapters
endpoint.
async function getMangaChapters(id, page = 1) {
const url = `https://api.jikan.moe/v3/manga/${id}/chapters/${page}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
getMangaChapters(13, 1)
.then((data) => console.log(data))
.catch((error) => console.log(error));
Conclusion
In this blog, we have explored how to use Jikan API with JavaScript. We have covered different endpoints and their usage with example code. You can use this API to create different anime and manga related applications.