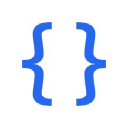
JSONbin.io
DevelopmentFree JSON storage service. Ideal for small scale Web apps, Websites and Mobile apps
π Documentation & Examples
Everything you need to integrate with JSONbin.io
π Quick Start Examples
// JSONbin.io API Example
const response = await fetch('https://jsonbin.io', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
JSONBin is a free JSON storage service tailored for developers seeking a reliable solution for their small-scale web applications, websites, and mobile apps. With its simple interface and seamless integration capabilities, JSONBin allows users to effortlessly store, retrieve, and manage JSON data. Whether you're launching a new project or need a quick solution to handle JSON in your applications, JSONBin provides the flexibility and functionality necessary to keep your data organized and accessible. The service is ideal for developers who prefer a lightweight yet powerful API that supports the dynamic needs of modern web and mobile platforms.
Utilizing JSONBin comes with numerous benefits that enhance productivity and streamline development processes. Key advantages include:
- Free and easy-to-use service for quick JSON data storage.
- Simplified API interface that accelerates integration into various applications.
- Real-time data retrieval, ensuring your applications have the latest information.
- Secure storage options that protect your data with user authentication.
- Excellent for prototyping, testing, and deploying small-scale applications without infrastructure overhead.
Here is a JavaScript code example for using the JSONBin API to store and retrieve data:
const axios = require('axios');
// Define the API endpoint
const apiUrl = 'https://api.jsonbin.io/v3/b';
// Store data
async function storeData(data) {
try {
const response = await axios.post(apiUrl, data, {
headers: {
'Content-Type': 'application/json',
'X-Master-Key': 'YOUR_MASTER_KEY',
},
});
console.log('Data stored successfully:', response.data);
} catch (error) {
console.error('Error storing data:', error);
}
}
// Retrieve data
async function getData(binId) {
try {
const response = await axios.get(`${apiUrl}/${binId}`, {
headers: {
'X-Bin-Version-Rev': 'latest',
},
});
console.log('Data retrieved successfully:', response.data);
} catch (error) {
console.error('Error retrieving data:', error);
}
}
// Example usage
const exampleData = {
name: 'John Doe',
email: 'john.doe@example.com',
};
storeData(exampleData);
getData('YOUR_BIN_ID');
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes