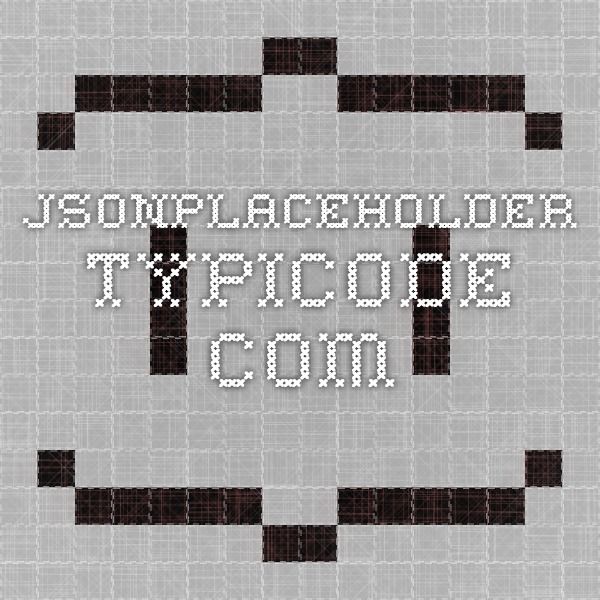
JSONPlaceholder
DevelopmentIntroduction
JSONPlaceholder is a free online REST API that delivers fake data in JSON format. The API is designed for developers who are building web and mobile applications and need access to mock data to test their applications or create demo sites.
In this blog post, we will explore the various endpoints available in JSONPlaceholder API and provide examples of how to consume the API using JavaScript.
Getting Started
To use the JSONPlaceholder API, you first need to familiarize yourself with the endpoints. The API has several endpoints, each with its own unique URL and data.
Basic Usage
To access an endpoint, simply make an HTTP request to the endpoint URL. The data returned by the endpoint will be in JSON format. Here is an example of how to retrieve data from the /posts endpoint using JavaScript:
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we're using the fetch
API to make a GET request to the /posts
endpoint. Once the data is retrieved, we're parsing it using the json()
method and logging it to the console. If an error occurs during the request, we're logging the error to the console as well.
You can also use XMLHttpRequest
to make an HTTP request. Here's an alternate example of how to retrieve data from the /posts endpoint using XMLHttpRequest
:
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://jsonplaceholder.typicode.com/posts');
xhr.onload = function() {
if (xhr.status === 200) {
console.log(JSON.parse(xhr.responseText));
} else {
console.error('Error:', xhr.statusText);
}
};
xhr.onerror = function() {
console.error('Error:', xhr.statusText);
};
xhr.send();
In this example, we're creating a new instance of XMLHttpRequest
and opening a GET request to the /posts
endpoint. Once the data is retrieved, we're parsing it using the JSON.parse()
method and logging it to the console. If an error occurs during the request, we're logging the error to the console as well.
Endpoints
Here are some of the most commonly used endpoints in the JSONPlaceholder API.
/posts
The /posts
endpoint returns a list of blog posts. Here's an example of how to retrieve data from the /posts
endpoint:
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
/comments
The /comments
endpoint returns a list of comments made on blog posts. Here's an example of how to retrieve data from the /comments
endpoint:
fetch('https://jsonplaceholder.typicode.com/comments')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
/albums
The /albums
endpoint returns a list of albums. Here's an example of how to retrieve data from the /albums
endpoint:
fetch('https://jsonplaceholder.typicode.com/albums')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
/photos
The /photos
endpoint returns a list of photos. Here's an example of how to retrieve data from the /photos
endpoint:
fetch('https://jsonplaceholder.typicode.com/photos')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
/todos
The /todos
endpoint returns a list of todo items. Here's an example of how to retrieve data from the /todos
endpoint:
fetch('https://jsonplaceholder.typicode.com/todos')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
/users
The /users
endpoint returns a list of users. Here's an example of how to retrieve data from the /users
endpoint:
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
In this blog post, we explored the JSONPlaceholder API and provided examples of how to consume the API using JavaScript. We looked at the various endpoints available and demonstrated how to retrieve data from each endpoint. With this knowledge, you should be able to start using the JSONPlaceholder API to test your application or create demo sites.