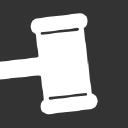
Judge0 CE
ProgrammingThe Online Code Execution System API is designed to provide seamless and efficient code execution capabilities. This powerful API allows developers to run code snippets in various programming languages in real-time, making it an invaluable tool for educational platforms, coding competitions, and online IDEs. With its easy-to-use interface, users can send code for execution, receive immediate feedback, and handle outputs effortlessly. The documentation can be found at https://ce.judge0.com/, where developers can explore detailed guides on utilizing the API to its full potential, including language support, execution parameters, and more.
Using the Online Code Execution System API comes with numerous advantages. Here are five benefits of integrating this API into your applications:
- Supports multiple programming languages for versatile code execution.
- Provides real-time feedback and output to enhance user experience.
- Easy integration with existing platforms for quick deployment.
- Scalable infrastructure that can handle high volumes of requests.
- Cost-effective solution compared to building a custom code execution environment.
Here’s a simple JavaScript code example for calling the API:
const axios = require('axios');
const runCode = async () => {
const url = 'https://api.judge0.com/submissions';
const code = `
#include <stdio.h>
int main() {
printf("Hello, World!\\n");
return 0;
}`;
const payload = {
source_code: Buffer.from(code).toString('base64'),
language_id: 4, // C Language
stdin: ""
};
try {
const response = await axios.post(url, payload);
const submissionId = response.data.token;
// Check the status of the submission
const resultResponse = await axios.get(`${url}/${submissionId}`);
console.log(resultResponse.data);
} catch (error) {
console.error('Error executing code:', error);
}
};
runCode();