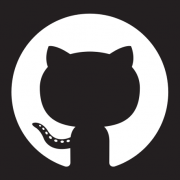
LCBO API
Food & DrinkLCBO API: A Guide to Public API Documentation in JavaScript
If you are a developer looking for data about the products sold by the LCBO, the Liquor Control Board of Ontario, you can access the public data through the LCBO API. This API can provide you with information on a vast range of alcoholic beverages including their names, prices, availability, locations, and much more.
In this guide, we will walk you through the process of accessing the API and provide you with some examples of how to retrieve data using JavaScript.
Accessing the API
Before you can start using the LCBO API, you'll need to get an API key. This key will allow you to access the resources that the LCBO API provides.
Getting Your API Key
To get your API key:
- Go to the LCBO API website: https://github.com/heycarsten/lcbo-api
- From the GitHub repository, click the "API Reference" button to view the API documentation.
- Click the "Get Your API Key" button to sign up for an API key.
- Fill out the registration form and submit it.
- Check your email inbox for a confirmation email. Follow the instructions to activate your API key.
Now that you have your API key, you can start using the LCBO API.
Retrieving Data Using JavaScript
To retrieve data from the LCBO API using JavaScript, you'll need to make an HTTP GET request to the appropriate endpoint using your API key as an authentication token.
Here's an example of how to retrieve a list of product categories:
const apiEndpoint = "https://lcboapi.com/products";
const apiKey = "YOUR_API_KEY";
const productCategoryParams = {
access_key: apiKey,
per_page: 100,
page: 1,
where_not: "is_dead",
order: "name.asc",
};
fetch(`${apiEndpoint}?${new URLSearchParams(productCategoryParams)}`)
.then((response) => response.json())
.then((json) => {
console.log(json);
})
.catch((error) => console.error(error));
In this example:
- We define the endpoint URL and our API key.
- We define an object called
productCategoryParams
that contains the parameters for our API request. - We use the
fetch
method to make the API request with the parameters. - We parse the response from the API as JSON using the
json
method. - We log the JSON response to the console.
You can modify the productCategoryParams
object to change the parameters you want to pass to the API endpoint. Refer to the LCBO API documentation for a full list of available parameters.
Conclusion
That's it for this guide on how to use the LCBO API in JavaScript. We hope these examples helped you get started! Remember to always refer to the API documentation for the full list of available resources and parameters.