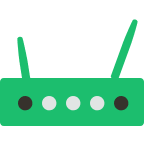
MAC address vendor lookup
DevelopmentHow to use macaddress.io API with JavaScript
Macaddress.io provides a public API to lookup MAC addresses and retrieve information about devices. In this blog post, we will explore how to use the API with JavaScript.
Getting started
Before you can use the macaddress.io API, you need to obtain an API key. You can sign up for a free account on their website and obtain the API key by following the instructions provided.
Once you have the API key, you can use it to make requests to the API using JavaScript.
Example code
Here are some example code snippets that demonstrate how to use the macaddress.io API with JavaScript.
Lookup vendor information for a MAC address
const api_key = 'your-api-key'
const mac_address = '00:0a:95:9d:68:16'
fetch(`https://api.macaddress.io/v1?apiKey=${api_key}&output=json&search=${mac_address}`)
.then(response => response.json())
.then(data => {
console.log(data.vendorDetails.companyName)
})
In this code snippet, we use the fetch
API to make a request to the macaddress.io API endpoint. We pass in our API key and the MAC address we want to lookup vendor information for.
The response from the API is returned as JSON data. We parse the JSON data and log the vendor company name to the console.
Lookup MAC address vendor information using Promise
const api_key = 'your-api-key'
const mac_address = '00:0a:95:9d:68:16'
function getVendorDetails(mac_address) {
return new Promise((resolve, reject) => {
fetch(`https://api.macaddress.io/v1?apiKey=${api_key}&output=json&search=${mac_address}`)
.then(response => response.json())
.then(data => {
resolve(data.vendorDetails)
})
.catch(error => {
reject(error)
})
})
}
getVendorDetails(mac_address)
.then(vendorDetails => {
console.log(vendorDetails.companyName)
})
.catch(error => {
console.error(error)
})
This code snippet demonstrates how to use the macaddress.io API with JavaScript async/await by wrapping the API call within a promise.
We define a function called getVendorDetails
that takes a MAC address as an argument. Inside the function, we make a request to the macaddress.io API endpoint using the fetch
API. We then parse the JSON data and resolve the vendor details.
We call the getVendorDetails
function with the MAC address to lookup and use the then
method to log the company name to the console.
Conclusion
Using the macaddress.io API with JavaScript is straightforward. You simply need to obtain an API key, make a request to the API endpoint with the key, and parse the JSON data returned by the endpoint.
We hope this blog post has been helpful in getting you started with the macaddress.io API using JavaScript. For more information on the API, you can refer to their official documentation.