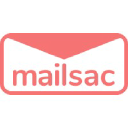
Mailsac
Test DataThe Disposable Email API, offered by Mailsac, has been specifically crafted to simplify the handling of temporary, disposable, and fake emails. Holding an essential role in the automation testing and development landscape, this API saves developers' valuable time and resources by eliminating the need to deal with spam and unreliable email addresses. With an easy-to-access documentation available at Mailsac's Website, utilizing the Disposable Email API can make email handling a breeze, letting you focus on more important aspects of your development project.
What sets this API apart is its comprehensive feature set that extends beyond simple disposable email creation. With facades for messages manipulation, email validation and even specific integrations with WebSocket for real-time email retrieval, the Disposable Email API provides a holistic solution to dealing with temporary emails. As a REST-based API, it supports standard HTTP methods, which makes it accessible from most modern technology stacks.
Key benefits of using the Disposable Email API are:
- Reduction in unwanted emails and spams
- Efficiency in email address validation during user registration
- Facilitation of testing and development procedures related to emails
- Instant delivery of emails with WebSocket integrations
- A REST API that is compatible with most modern programming platforms
Here is a JavaScript code example demonstrating how to call the API to fetch a list of all messages for a given email:
var request = require('request');
var options = {
url: 'https://api.mailsac.com/disposable-email/messages',
method: 'GET',
headers: {
'Mailsac-Key': 'YOUR_API_KEY'
},
qs: {
'email': 'example@mailsac.com'
}
};
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
console.log(body);
}
});
Ensure to replace 'YOUR_API_KEY'
with your actual API key and 'example@mailsac.com'
with the email address you wish to fetch messages from. This code uses the request
library to send a GET request to the API endpoint and then logs the response body.