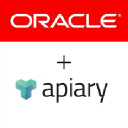
Mailtrap
EmailMailtrap provides a secure solution for testing emails during the development and staging phases, ensuring that developers can safely validate email functionalities without the risk of sending test messages to actual users. This service allows developers to capture and analyze outgoing email communications in a controlled environment, preventing spam and maintaining user trust. By integrating Mailtrap into your workflow, you can easily check how your emails will appear across different clients and devices, streamline the debugging process, and enhance overall email delivery quality.
Using the Mailtrap API comes with numerous advantages. Some of the key benefits include:
- Securely test emails without impacting real user experiences.
- Access to a user-friendly dashboard for managing and viewing emails.
- Detailed logging and reporting for easy debugging and analysis.
- Seamless integration with popular development frameworks and languages.
- Support for various email protocols, ensuring flexibility and compatibility.
Here's an example of how to use the Mailtrap API in JavaScript:
const fetch = require('node-fetch');
const sendTestEmail = async () => {
const response = await fetch('https://api.mailtrap.io/api/v1/inboxes/YOUR_INBOX_ID/send', {
method: 'POST',
headers: {
'Authorization': 'Bearer YOUR_API_TOKEN',
'Content-Type': 'application/json'
},
body: JSON.stringify({
from: { email: 'test@example.com', name: 'Test Sender' },
to: [{ email: 'recipient@example.com', name: 'Recipient' }],
subject: 'Test Email Subject',
html: '<h1>Hello World</h1><p>This is a test email from Mailtrap.</p>'
})
});
if (response.ok) {
const data = await response.json();
console.log('Test email sent successfully:', data);
} else {
console.error('Error sending test email:', response.status, response.statusText);
}
};
// Call the function to send the email
sendTestEmail();