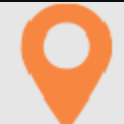
MapmyIndia
GeocodingUsing the MapMyIndia API with JavaScript
MapMyIndia is a popular mapping website that provides a range of APIs for developers to integrate maps, geolocation, and other location-based services into their applications. In this article, we will explore some of the most useful MapMyIndia APIs and show you how to use them with JavaScript.
Getting Started with the MapMyIndia API
To get started, you need to sign up for a MapMyIndia account. Once you have an account, you can access the MapMyIndia developer dashboard to get your API key.
var API_KEY = 'YOUR_API_KEY_HERE'
After getting your API key, you can start using the MapMyIndia API.
MapMyIndia Maps API
The MapMyIndia Maps API is one of the most powerful APIs the platform offers. It can be used to display interactive maps on your website or application, including the ability to add markers, overlays, and more.
Displaying a Map
To display a MapMyIndia map, you can use the MapMyIndia Map JavaScript library.
<!DOCTYPE html>
<html>
<head>
<title>MapMyIndia Map Example</title>
<script src='https://apis.mapmyindia.com/advancedmaps/api/{YOUR_API_KEY}/map_sdk.min.js'></script>
</head>
<body>
<div id='map' style='height: 400px;'></div>
<script>
var map = new MapmyIndia.Map('map', { center:[28.6139, 77.209], zoomControl: true });
</script>
</body>
</html>
This example creates a new MapMyIndia map centered on New Delhi, with the zoom control enabled.
Adding Markers
To add a marker to a MapMyIndia map, you can use the following code.
var marker = new MapmyIndia.Marker({
position: [28.6139, 77.209],
map: map,
title: 'New Delhi'
});
This will add a marker to the map at the specified coordinates.
MapMyIndia Geo-Coding API
The MapMyIndia Geo-Coding API allows you to convert a location (such as an address or place name) into geographic coordinates (latitude and longitude). This is useful when you need to display a location on a map, or when you want to perform location-based searches.
Geo-Coding an Address
To geocode an address, you can use the following code.
var address = '1600 Amphitheatre Parkway, Mountain View, CA';
var geocoder = new MapmyIndia.Geocoder(API_KEY);
geocoder.getLatLng(address, function(data) {
var latLng = [data.results[0].lat, data.results[0].lng];
console.log(latLng);
});
This example geocodes the address of the Googleplex in Mountain View, California, and logs the resulting coordinates to the console.
Conclusion
MapMyIndia offers a range of powerful APIs that can be used with JavaScript to create interactive maps, geocode addresses, and perform location-based searches. With these tools, you can add powerful location-based features to your applications and provide a better user experience for your users.