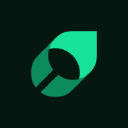
Mintlify
ProgrammingThe API for programmatically generating documentation for code is an essential tool for developers aiming to streamline their documentation process. This API simplifies the creation of comprehensive, easily navigable documentation directly from codebases, enhancing productivity and ensuring that documentation remains up-to-date with the latest developments. It provides a powerful solution for projects of all sizes, allowing teams to focus more on code development and less on the often tedious task of writing and maintaining documentation. For detailed information and guidance on implementation, you can visit the official documentation at Mintlify API Docs.
Utilizing this API comes with numerous benefits that can significantly improve your development workflow. Key advantages include:
- Automated documentation generation saves time and effort.
- Ensures consistency and accuracy across all documentation.
- Easy integration into existing development processes or tools.
- Supports multiple programming languages and frameworks.
- Enhances collaboration among team members by providing clear and organized documentation.
Here's a JavaScript code example demonstrating how to call the API:
const axios = require('axios');
const generateDocumentation = async (code) => {
try {
const response = await axios.post('https://api.mintlify.com/generate-docs', {
body: code,
language: 'javascript'
});
console.log('Documentation generated successfully:', response.data);
} catch (error) {
console.error('Error generating documentation:', error);
}
};
// Example usage
generateDocumentation(`
function greet(name) {
return 'Hello, ' + name + '!';
}
`);