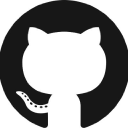
Movebank
AnimalsExplore the Movebank Public API with JavaScript
Movebank is a free, online platform for storing, sharing, analyzing, and publishing animal tracking data. The Movebank Public API allows developers to access data about animal movements, including tracking data, environmental data, and behavioral observations.
This API is a RESTful web service that uses HTTP requests to GET, POST, PUT, and DELETE data. The API returns data in JSON format, which makes it easy to work with in JavaScript.
Getting Started
To use the Movebank Public API, you need to create an account and get an API key. You can do this by visiting the Movebank API documentation at https://github.com/movebank/movebank-api-doc.
Once you have an API key, you can start making requests to the API using JavaScript. Here are some examples:
Example 1: Get all studies
To get a list of all studies available in Movebank, you can use the following JavaScript code:
fetch('https://api.movebank.org/v2/studies?&access_token=YOUR_API_KEY')
.then(response => response.json())
.then(data => console.log(data));
This code sends a GET request to the https://api.movebank.org/v2/studies
endpoint with your API key as a query parameter. It then logs the response data to the console.
Example 2: Get all animals in a study
To get a list of all animals in a specific study, you can use the following JavaScript code:
fetch('https://api.movebank.org/v2/study-ids/YOUR_STUDY_ID/individuals?&access_token=YOUR_API_KEY')
.then(response => response.json())
.then(data => console.log(data));
This code sends a GET request to the https://api.movebank.org/v2/study-ids/YOUR_STUDY_ID/individuals
endpoint with your API key and the ID of the study you want to get data for as query parameters. It then logs the response data to the console.
Example 3: Get location data for an animal
To get location data for a specific animal in a study, you can use the following JavaScript code:
fetch('https://api.movebank.org/v2/individuals/YOUR_INDIVIDUAL_ID/locations?start_date=2020-01-01&end_date=2020-01-31&access_token=YOUR_API_KEY')
.then(response => response.json())
.then(data => console.log(data));
This code sends a GET request to the https://api.movebank.org/v2/individuals/YOUR_INDIVIDUAL_ID/locations
endpoint with your API key, the ID of the animal you want to get data for, and the start and end dates for the data you want. It then logs the response data to the console.
Conclusion
The Movebank Public API is a powerful tool for accessing data about animal movements. With JavaScript, you can easily make requests to the API and work with the response data in your web applications. This guide has provided some basic examples of how to use the API with JavaScript, but there are many more endpoints and parameters available in the API documentation. So, go ahead and explore more!