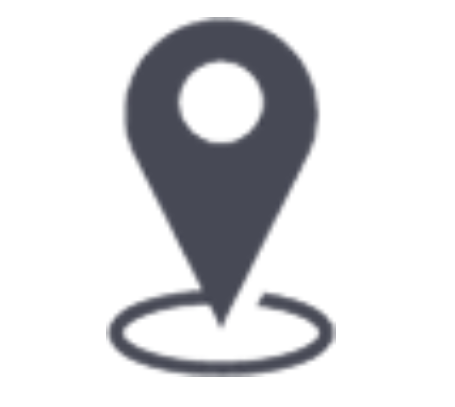
Native Land
GeocodingThe Native Land API, available on their website, provides geocoding information for Indigenous territories, languages, and treaties. With this API, you can easily access information about the Indigenous territories and languages that occupy a specific area, as well as any relevant treaties or agreements. Whether you're a researcher, educator, or just someone who wants to learn more about the Indigenous peoples of a specific region, the Native Land API is a valuable resource that can help you better understand the land you live on. So why not check it out today and start exploring the rich history and culture of Indigenous peoples around the world?
📚 Documentation & Examples
Everything you need to integrate with Native Land
🚀 Quick Start Examples
// Native Land API Example
const response = await fetch('https://native-land.ca/resources/api-docs/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
How to Use Native Land API Docs
Native Land is a website that offers an API service that allows our users to get information about Indigenous territories, languages, and treaties all around the world. Our API is designed to be accessible and user-friendly to developers of all levels of experience. In this blog post, we will explore how to use the Native Land API docs and how to get the most out of the available API endpoints.
Available API Endpoints
The Native Land API offers a wide range of different endpoints that allow you to retrieve location data, territory data, treaty data, and language data. We provide a comprehensive list of available endpoints on the Native Land API documentation website.
API Example Code in JavaScript
Here are some example codes that show you how to use the Native Land API in JavaScript. You can use these examples as a starting point to build your own custom application that utilizes the Native Land API.
Retrieving Territory Data
const fetchTerritoryData = async () => {
const response = await fetch('https://native-land.ca/api/v1/territories/');
const data = await response.json();
console.log(data);
}
This example retrieves all available territory data from the Native Land API. The fetch()
method is used to send a GET
request, and the received response is then converted into JSON format. The data retrieved is then logged to the console.
Retrieving Treaty Data
const fetchTreatyData = async () => {
const response = await fetch('https://native-land.ca/api/v1/treaties/');
const data = await response.json();
console.log(data);
}
This example retrieves all available treaty data from the Native Land API. The fetch()
method is used to send a GET
request, and the received response is then converted into JSON format. The data retrieved is then logged to the console.
Retrieving Language Data
const fetchLanguageData = async () => {
const response = await fetch('https://native-land.ca/api/v1/languages/');
const data = await response.json();
console.log(data);
}
This example retrieves all available language data from the Native Land API. The fetch()
method is used to send a GET
request, and the received response is then converted into JSON format. The data retrieved is then logged to the console.
Retrieving Location Data
const fetchLocationData = async (latitude, longitude) => {
const response = await fetch(`https://native-land.ca/api/v1/position/${latitude}/${longitude}/`);
const data = await response.json();
console.log(data);
}
This example retrieves location-based data from the Native Land API. The fetch()
method is used to send a GET
request with the latitude and longitude values passed in as parameters. The received response is then converted into JSON format, and the data retrieved is logged to the console.
Conclusion
The Native Land API provides a unique and accessible service for developers looking to incorporate Indigenous land data into their applications. With the available API endpoints and example codes in JavaScript, developers can quickly and easily retrieve and use the data they need from the Native Land API in their applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes