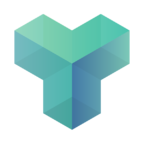
Nexchange
CryptocurrencyPublic API for Nexchange2
Nexchange2 is a cryptocurrency exchange that provides APIs to their users to access the functionalities of the exchange programmatically. In this blog post, we will be discussing the various endpoints available in the Nexchange2 public API.
To use the API, you need to first obtain an API key from the Nexchange2 website. Once you have the API key, you can use it to make requests to the API endpoints.
All API requests should be made to https://api.nexchange2.io/
with the endpoint being appended to the end of the URL. Data should be submitted in JSON format.
Available endpoints
Get available markets
Use this endpoint to get the list of available trading pairs in the Nexchange2 exchange.
async function getMarkets() {
const response = await fetch('https://api.nexchange2.io/market/');
const markets = await response.json();
return markets;
}
Get market ticker
Use this endpoint to get the ticker data for a specific trading pair.
async function getTicker(market) {
const response = await fetch(`https://api.nexchange2.io/market/${market}/ticker/`);
const tickerData = await response.json();
return tickerData;
}
Get market depth
Use this endpoint to get the order book depth for a specific trading pair.
async function getDepth(market) {
const response = await fetch(`https://api.nexchange2.io/market/${market}/depth/`);
const depthData = await response.json();
return depthData;
}
Get market trades
Use this endpoint to get the recent trades for a specific trading pair.
async function getTrades(market) {
const response = await fetch(`https://api.nexchange2.io/market/${market}/trades/`);
const trades = await response.json();
return trades;
}
Get market OHLCV
Use this endpoint to get the OHLCV (open, high, low, close, volume) data for a specific trading pair.
async function getOHLCV(market, interval) {
const response = await fetch(`https://api.nexchange2.io/market/${market}/ohlcv/?interval=${interval}`);
const ohlcvData = await response.json();
return ohlcvData;
}
Conclusion
In this blog post, we have explored the various endpoints available in the Nexchange2 public API. With these endpoints, you can programmatically access the functionalities of the exchange and build your own cryptocurrency trading bots and applications.