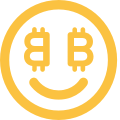
📚 Documentation & Examples
Everything you need to integrate with NiceHash
🚀 Quick Start Examples
// NiceHash API Example
const response = await fetch('https://docs.nicehash.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Public API Docs for NiceHash
NiceHash is a marketplace for mining hash power. The platform offers a public API that allows users to interact with various features of the platform programmatically. In this article, we will explore some examples of using the NiceHash public API in JavaScript.
Getting Started
Before we dive into the examples, it is important to note that NiceHash requires all API requests to be authenticated with an API key. To get started, you will need to sign up for a NiceHash account and generate an API key. You can do this by following the instructions on the NiceHash website.
Once you have your API key, you can use it to authenticate your requests to the NiceHash API. All API endpoints are accessed via HTTP requests and the responses are returned in JSON format.
Example API Calls
Retrieving Your Account Balance
To retrieve your account balance, you can use the following code:
const apiKey = 'your_api_key';
fetch('https://api.nicehash.com/api?method=balance&id=0&key=' + apiKey)
.then(response => response.json())
.then(data => {
console.log(`Your current balance is: ${data.result.balance}`);
})
.catch(error => console.error(error));
Retrieving Your Mining Rig Information
To retrieve information about your mining rigs, you can use the following code:
const apiKey = 'your_api_key';
fetch('https://api.nicehash.com/api?method=rigs2&id=0&key=' + apiKey)
.then(response => response.json())
.then(data => {
console.log(`You have ${data.result.length} mining rigs.`);
data.result.forEach(rig => {
console.log(`Rig name: ${rig.name}, status: ${rig.alive ? "alive" : "offline"}`);
});
})
.catch(error => console.error(error));
Retrieving Market Information
To retrieve information about the NiceHash marketplace, you can use the following code:
const apiKey = 'your_api_key';
fetch('https://api.nicehash.com/api?method=simplemultialgo.info&id=0&key=' + apiKey)
.then(response => response.json())
.then(data => {
console.log(`There are ${data.result.length} market algorithms available.`);
data.result.forEach(algo => {
console.log(`${algo.name}: ${algo.port}`);
});
})
.catch(error => console.error(error));
Conclusion
In this article, we explored some examples of using the NiceHash public API in JavaScript. We looked at how to retrieve your account balance, your mining rig information, and market information. By using these examples as a starting point, you can begin to integrate the NiceHash API into your own applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes