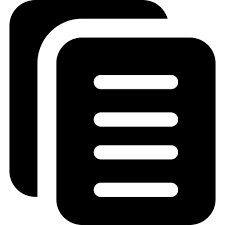
Nominatim
GeocodingHow to Use the Nominatim Public API in JavaScript
The Nominatim Public API is a powerful tool for geocoding and reverse geocoding, and it is now easier than ever to use in JavaScript. In this blog post, we will provide you with all the necessary information to get started.
Getting Started
First, you will need to obtain an API key. You can do this by visiting the Nominatim Public API website and creating an account. Once you have an API key, you can begin making requests.
Making a Request
The Nominatim Public API is a RESTful API, meaning that you can make requests using HTTP methods such as GET and POST. Here is an example of how to make a request using JavaScript:
fetch("https://nominatim.org/release-docs/latest/api/search?q=New+York&format=json")
.then(response => response.json())
.then(data => console.log(data));
In this example, we are using the fetch
method to send a GET request to the API endpoint. The q
parameter specifies the query string and the format
parameter specifies the response format.
Once the API returns a response, we are using the json
method to convert the response body to a JavaScript object. Finally, we are logging the data to the console.
Parameters
The Nominatim Public API supports a wide range of parameters that you can use to customize your requests. Here is an example of how to include parameters in your request:
const params = new URLSearchParams({
q: "New York",
format: "json",
limit: 10,
});
fetch(`https://nominatim.org/release-docs/latest/api/search?${params}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are using the URLSearchParams
class to create a new URL object containing our query string parameters. We are then passing this object to the API endpoint using the ${params}
syntax.
Conclusion
In this blog post, we have shown you how to use the Nominatim Public API in JavaScript. We hope that this information will be useful to you and that you will be able to use this powerful tool to create amazing geocoding and reverse geocoding applications.