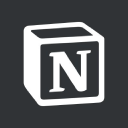
Notion
Documents & ProductivityThe Notion API provides a robust solution for developers looking to integrate their applications with Notion's versatile workspace functionalities. By leveraging this API, users can access and manipulate data within Notion, allowing seamless interaction with pages, databases, and blocks. The official documentation available at Notion Developer Docs serves as a comprehensive guide, offering insights into authentication, API endpoints, and best practices. This integration unlocks the potential for automating tasks, enhancing workflows, and creating custom applications tailored to specific business needs, ultimately improving productivity and collaboration across teams.
Key benefits of utilizing the Notion API include:
- Enhanced Automation: Streamline repetitive tasks by automating data entry and updates.
- Custom Workflows: Create personalized workflows that align with your team's processes and project management requirements.
- Unified Data Management: Consolidate data from various sources into a centralized Notion database for easy access and organization.
- Real-time Collaboration: Facilitate team collaboration by enabling live updates and information sharing across Notion pages.
- Extensibility: Expand the functionality of Notion by integrating with other applications and services, tailoring solutions to meet unique organizational needs.
Here's a simple JavaScript code example to call the Notion API:
const fetch = require('node-fetch');
const NOTION_API_URL = 'https://api.notion.com/v1/pages';
const NOTION_API_KEY = 'your_notion_integration_token';
async function createNotionPage() {
const response = await fetch(NOTION_API_URL, {
method: 'POST',
headers: {
'Authorization': `Bearer ${NOTION_API_KEY}`,
'Content-Type': 'application/json',
'Notion-Version': '2022-06-28'
},
body: JSON.stringify({
parent: { database_id: 'your_database_id' },
properties: {
Name: {
title: [
{
text: {
content: 'New Page Title'
}
}
]
}
}
})
});
if (response.ok) {
const data = await response.json();
console.log('Page created successfully:', data);
} else {
console.error('Error creating page:', response.statusText);
}
}
createNotionPage();