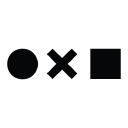
Noun Project API
DevelopmentUsing the Noun Project API with JavaScript
The Noun Project is a website that provides a vast collection of icons and symbols that can be used for various purposes. With its API, developers can integrate its high-quality icons and symbols into their projects easily. In this tutorial, we will show you how to use the Noun Project API with JavaScript.
Getting Started
First, you need to sign up for a free account on the Noun Project website. Then, you need to create an API key, which you can do by going to https://thenounproject.com/developers/apps/new.
After creating your API key, you can use it to make requests to the API endpoints. All API endpoints require an Access Token, which can be retrieved by making a POST request to the https://api.thenounproject.com/oauth/access_token endpoint.
In JavaScript, you can use the XMLHttpRequest or Fetch API to make HTTP requests to the API endpoints. Here's an example of how to retrieve a list of all icons from the API:
const accessToken = "your_access_token";
const url = "https://api.thenounproject.com/icons";
fetch(url, {
headers: {
Authorization: `Bearer ${accessToken}`,
},
})
.then((res) => res.json())
.then((data) => console.log(data));
In this example, we're using the Fetch API to make a GET request to the https://api.thenounproject.com/icons endpoint. We're passing our Access Token in the Authorization header and parsing the response as JSON.
Searching for Icons
You can also search for icons using the Noun Project API. To search for icons, you need to make a GET request to the https://api.thenounproject.com/icons/{term} endpoint, where {term}
is the search term.
Here's an example of how to search for icons using the API:
const accessToken = "your_access_token";
const term = "search_term";
const url = `https://api.thenounproject.com/icons/${term}`;
fetch(url, {
headers: {
Authorization: `Bearer ${accessToken}`,
},
})
.then((res) => res.json())
.then((data) => console.log(data));
In this example, we're passing the search term in the URL and making a GET request to the endpoint. We're also passing our Access Token in the Authorization header.
Conclusion
In this tutorial, we've shown you how to use the Noun Project API with JavaScript. You can use this API to retrieve a list of icons or search for specific icons. You can also use this API to create your own custom icons and upload them to the Noun Project website.