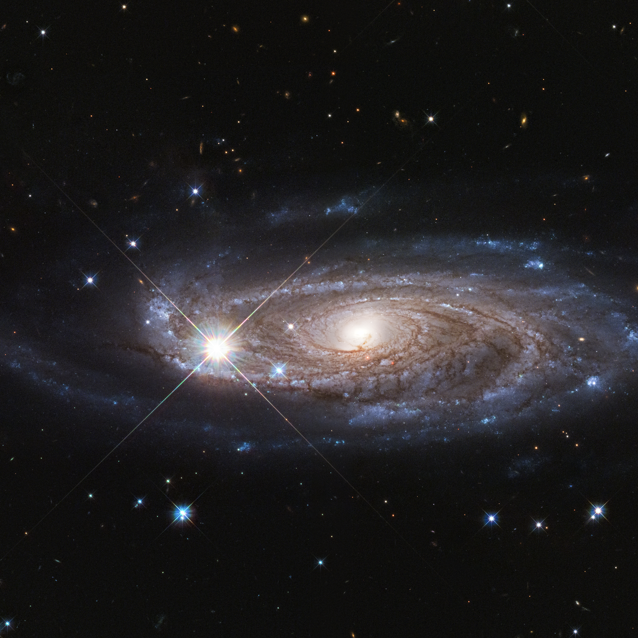
Open astronomy Catalogs API
Sports & FitnessIntroduction to OACAPI
OACAPI stands for Open Astronomy Catalogs API. It provides an interface to access public astronomy catalogs in a convenient way. In this blog, we will explore the usage of this API and see some examples of how to integrate it into your JavaScript projects.
Getting Started
To use the OACAPI, we need to first authenticate ourselves with an access token. You can get the token from the OACAPI team by contacting them. Once you have the access token, you can make requests to the API endpoints.
Example code
const access_token = "YOUR_ACCESS_TOKEN";
const getCatalogs = async () => {
const response = await fetch(
"https://api.astrocats.space/catalogs",
{
headers: {
Authorization: `Bearer ${access_token}`,
},
}
);
const catalogs = await response.json();
console.log(catalogs);
};
getCatalogs();
In the above example code, we're fetching the catalogs from the API endpoint https://api.astrocats.space/catalogs. We're passing the access token in the header as an authorization token.
Response
The response we get from the above request will be a list of catalogs with their details. Here's an example of the response.
[
{
"name": "SDSS-I/II Supernova Survey",
"description": "The SDSS-II Supernova Survey (SN Survey-II) was an extension of the SDSS-II Legacy Survey designed to detect a large number of Type Ia supernovae",
"url": "https://api.astrocats.space/sdss/",
"bibcode": "2008AJ....135..338F"
},
{
"name": "Master Lens Database",
"description": "A database of gravitational lensing",
"url": "https://api.astrocats.space/mlens/",
"bibcode": "2010A&A...523A..66T"
},
...
]
Conclusion
In this blog, we explored the OACAPI and how to make requests to its API endpoints using JavaScript. We also saw an example response from one of the endpoints. OACAPI is a powerful tool for accessing public astronomy catalogs, and we hope this blog will help you get started with using it in your own projects.