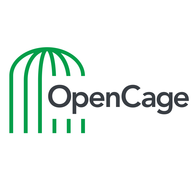
OpenCage
GeocodingOpenCageData API Overview
OpenCageData is a geocoding API service that helps to convert latitude and longitude coordinates into human-readable addresses and vice versa. It's a perfect tool for developers who need to implement location-based functionalities in their web or mobile applications. In this blog post, we will explore the OpenCageData API documentation and provide JavaScript code examples to show how to use this API for geocoding and reverse geocoding.
Getting Started
Before we can start using the OpenCageData API, we need to sign up for a free API key on their website. Once we have an API key, we can use it to make HTTP requests to the OpenCageData API.
Geocoding API
The geocoding API is used to convert a location, such as a city or an address, into latitude and longitude coordinates. Here's an example of how to use the OpenCageData geocoding API in JavaScript:
const API_KEY = "YOUR_API_KEY";
const location = "London, UK";
const url = `https://api.opencagedata.com/geocode/v1/json?q=${encodeURIComponent(
location
)}&key=${API_KEY}`;
fetch(url)
.then((response) => response.json())
.then((data) => {
const results = data.results;
if (results.length > 0) {
const coordinates = results[0].geometry;
console.log(coordinates);
} else {
console.log("No results found");
}
})
.catch((error) => console.error(error));
In this example, we first define our API_KEY
variable and the location
that we want to geocode. We then construct the API endpoint URL by encoding the location parameter with encodeURIComponent
. Finally, we make an HTTP request using the fetch
method, parse the response JSON, and log the resulting coordinates to the console.
Reverse Geocoding API
The reverse geocoding API is used to convert latitude and longitude coordinates into human-readable addresses. Here's an example of how to use the OpenCageData reverse geocoding API in JavaScript:
const API_KEY = "YOUR_API_KEY";
const latitude = 51.507222;
const longitude = -0.127758;
const url = `https://api.opencagedata.com/geocode/v1/json?q=${encodeURIComponent(
`${latitude},${longitude}`
)}&key=${API_KEY}`;
fetch(url)
.then((response) => response.json())
.then((data) => {
const results = data.results;
if (results.length > 0) {
const address = results[0].formatted;
console.log(address);
} else {
console.log("No results found");
}
})
.catch((error) => console.error(error));
In this example, we first define our API_KEY
variable and the latitude
and longitude
coordinates that we want to reverse geocode. We then construct the API endpoint URL by encoding the coordinates parameter with encodeURIComponent
. Finally, we make an HTTP request using the fetch
method, parse the response JSON, and log the resulting address to the console.
Conclusion
In this blog post, we have shown how to use the OpenCageData API for geocoding and reverse geocoding. We have provided JavaScript code examples that demonstrate how to make HTTP requests to the OpenCageData API using the fetch
method. With this knowledge, you can now integrate geocoding and reverse geocoding functionalities into your own web or mobile applications.