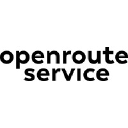
openrouteservice.org
GeocodingThe OpenRouteService API offers an extensive range of functionalities for developers looking to integrate geographic data and routing services into their applications. With capabilities such as directions, points of interest (POIs), isochrones, geocoding (including reverse geocoding), and elevation data, it provides a robust solution for a variety of location-based needs. Whether you're developing a navigation app, a travel planner, or a geographic analysis tool, this API delivers accurate and efficient routing and mapping information that enhances user experience and application performance. By leveraging state-of-the-art algorithms and a rich dataset, OpenRouteService empowers users to access critical location services seamlessly.
Using the OpenRouteService API can lead to significant improvements in application functionality and user engagement. Here are five compelling benefits of integrating this API into your projects:
- Access diverse location-based services from a single API
- Obtain real-time routing and navigation data to improve user experience
- Implement geocoding to easily convert addresses into geographical coordinates
- Utilize elevation data for enhanced geographical analyses and planning
- Easily retrieve points of interest to enrich your application's content and offerings
Here's a JavaScript code example for calling the OpenRouteService API to get directions:
const fetch = require('node-fetch');
const API_KEY = 'YOUR_API_KEY'; // Replace with your OpenRouteService API key
const url = 'https://api.openrouteservice.org/v2/directions/driving-car';
const getDirections = async (start, end) => {
const response = await fetch(`${url}?start=${start}&end=${end}`, {
method: 'GET',
headers: {
'Authorization': API_KEY,
'Content-Type': 'application/json'
}
});
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
return data.routes;
};
// Example usage making a request with coordinates
const start = '8.681495,49.414446'; // Start point coordinates (longitude, latitude)
const end = '8.687872,49.420318'; // End point coordinates (longitude, latitude)
getDirections(start, end)
.then(routes => console.log(routes))
.catch(error => console.error('Error fetching directions:', error));