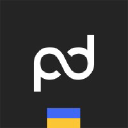
PandaDoc
Documents & ProductivityThe DocGen and eSignatures API is a powerful tool for businesses seeking to streamline their document generation and signing processes. With PandaDoc’s API, developers can easily integrate features that allow for the automated creation of documents and the management of eSignatures, enhancing productivity and ensuring compliance. This API provides a seamless experience for users by allowing them to generate professional-looking templates, manage workflows, and securely collect signatures—all within a robust platform that prioritizes user security and data integrity. The comprehensive documentation available at PandaDoc Developers ensures that developers can quickly get started and efficiently implement the API into their applications.
Utilizing the DocGen and eSignatures API brings numerous advantages for businesses and developers alike. Key benefits include:
- Enhanced automation of document workflows, reducing manual tasks and errors.
- Customizable document templates that cater to unique business needs.
- Secure eSignature functionality that ensures legal compliance and audit trails.
- Integration capabilities with popular CRMs and other software, enabling a seamless user experience.
- Comprehensive analytics tools that provide insights into document performance and customer engagement.
Here is a JavaScript code example for calling the DocGen and eSignatures API:
const axios = require('axios');
const apiKey = 'YOUR_API_KEY';
const url = 'https://api.pandadoc.com/v1/documents';
const generateDocument = async () => {
const documentData = {
name: 'Sample Document',
url: 'https://example.com/sample_template',
fields: {
recipient: {
email: 'recipient@example.com',
name: 'John Doe'
}
}
};
try {
const response = await axios.post(url, documentData, {
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
});
console.log('Document generated successfully:', response.data);
} catch (error) {
console.error('Error generating document:', error);
}
};
generateDocument();