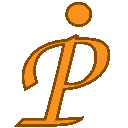
PhantAuth
DevelopmentIntroduction to PhantAuth API
PhantAuth is a public API that provides a user authentication service for various apps and websites. It offers a simple and secure way to manage user data and provides various authentication methods like Single Sign-On (SSO), passwordless authentication, social media login, and more.
In this blog post, we will explore the PhantAuth API and learn how to use it to authenticate users in our JavaScript applications.
Getting Started with PhantAuth
To use the PhantAuth API, you must first create an account on its website and obtain an API key. The API key is necessary for authenticating requests to the API. Once you obtain the API key, you can start using the PhantAuth API in your JavaScript applications.
PhantAuth API Endpoints
PhantAuth API exposes various endpoints that can be used to authenticate users and manage user data. Some of the essential endpoints are:
/api/v1/auth
: This is the main authentication endpoint that can be used to authenticate users using different authentication methods./api/v1/profile
: This endpoint can be used to retrieve user profiles./api/v1/applications
: This endpoint can be used to manage applications (add/edit/delete)./api/v1/accounts
: This endpoint can be used to manage user accounts (create/edit/delete).
Using PhantAuth API in JavaScript
To use the PhantAuth API in your JavaScript application, you can use the fetch()
method provided by the browser. Here is an example code snippet that authenticates a user using the PhantAuth API:
// Replace API_KEY with your API key obtained from PhantAuth website.
const API_KEY = "API_KEY";
fetch("https://www.phantauth.net/api/v1/auth", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${API_KEY}`,
},
body: JSON.stringify({
"provider": "email",
"email": "user@example.com",
"password": "password",
}),
})
.then((response) => {
if (response.ok) {
return response.json();
} else {
throw new Error("Failed to authenticate user");
}
})
.then((data) => {
console.log("User authenticated successfully", data);
})
.catch((error) => {
console.error(error);
});
This code snippet sends a POST request to the /api/v1/auth
endpoint with the user's email and password as credentials and obtains a JWT token if the credentials are correct. The JWT token can be used to authenticate further requests to the API.
Conclusion
PhantAuth is an easy-to-use authentication API that can be integrated into various web applications. It provides various authentication methods and exposes various endpoints to manage user data. In this blog post, we explored the basics of the PhantAuth API and learned how to use it in our JavaScript applications.