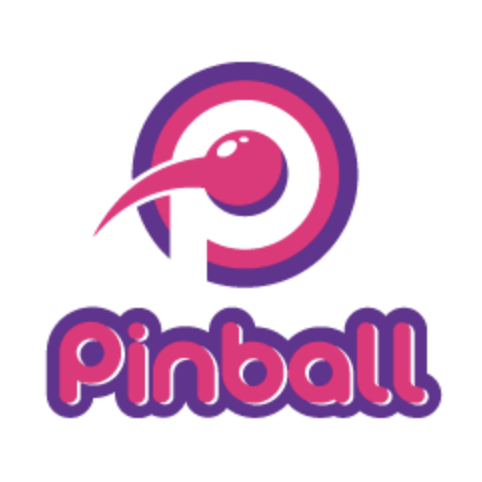
Pinball Map
Sports & FitnessUsing the Pinball Map API in JavaScript
Pinball Map provides a public API that allows developers to access a vast amount of data about pinball machines and related information. In this tutorial, we'll explore how to use the Pinball Map API in JavaScript to fetch data and display it on a webpage.
Getting Started
Before we start using the Pinball Map API, we'll need to obtain an API key. This can be done by visiting https://pinballmap.com/api/v1/signup and filling out the registration form. Once registered, you can obtain your API key by visiting your account settings.
Fetching data from the API
To fetch data from the Pinball Map API, we'll be making HTTP requests to various endpoints using JavaScript's fetch()
function. Here's an example of retrieving a list of locations near a specific latitude/longitude:
fetch('https://pinballmap.com/api/v1/locations/nearby?lat=45.5231&lon=-122.6765')
.then(response => response.json())
.then(data => {
console.log(data);
});
In the above example, we're making a GET request to the /api/v1/locations/nearby
endpoint with latitude and longitude parameters. We're then parsing the JSON response using the .json()
method and logging the data to the console.
Displaying data on a webpage
Once we've fetched data from the API, we can display it on a webpage by manipulating the DOM. Here's an example of using the above API call to display a list of locations on a webpage:
<!DOCTYPE html>
<html>
<head>
<title>Pinball Locations</title>
</head>
<body>
<ul id="locations"></ul>
<script>
fetch('https://pinballmap.com/api/v1/locations/nearby?lat=45.5231&lon=-122.6765')
.then(response => response.json())
.then(data => {
const locationsList = document.getElementById('locations');
data.forEach(location => {
const li = document.createElement('li');
li.textContent = location.name;
locationsList.appendChild(li);
});
});
</script>
</body>
</html>
In the above example, we're creating a basic HTML page with an empty unordered list (<ul>
) element. We're then using the above API call to fetch locations near a latitude and longitude, and creating a list item (<li>
) element for each location returned, and appending it to the unordered list.
Conclusion
Using the Pinball Map API in JavaScript is a powerful way to fetch and display data about pinball machines and locations. With just a few lines of code, we were able to retrieve data from the API and display it on a webpage. For more information about the Pinball Map API, check out the official documentation at https://pinballmap.com/api/v1/docs.