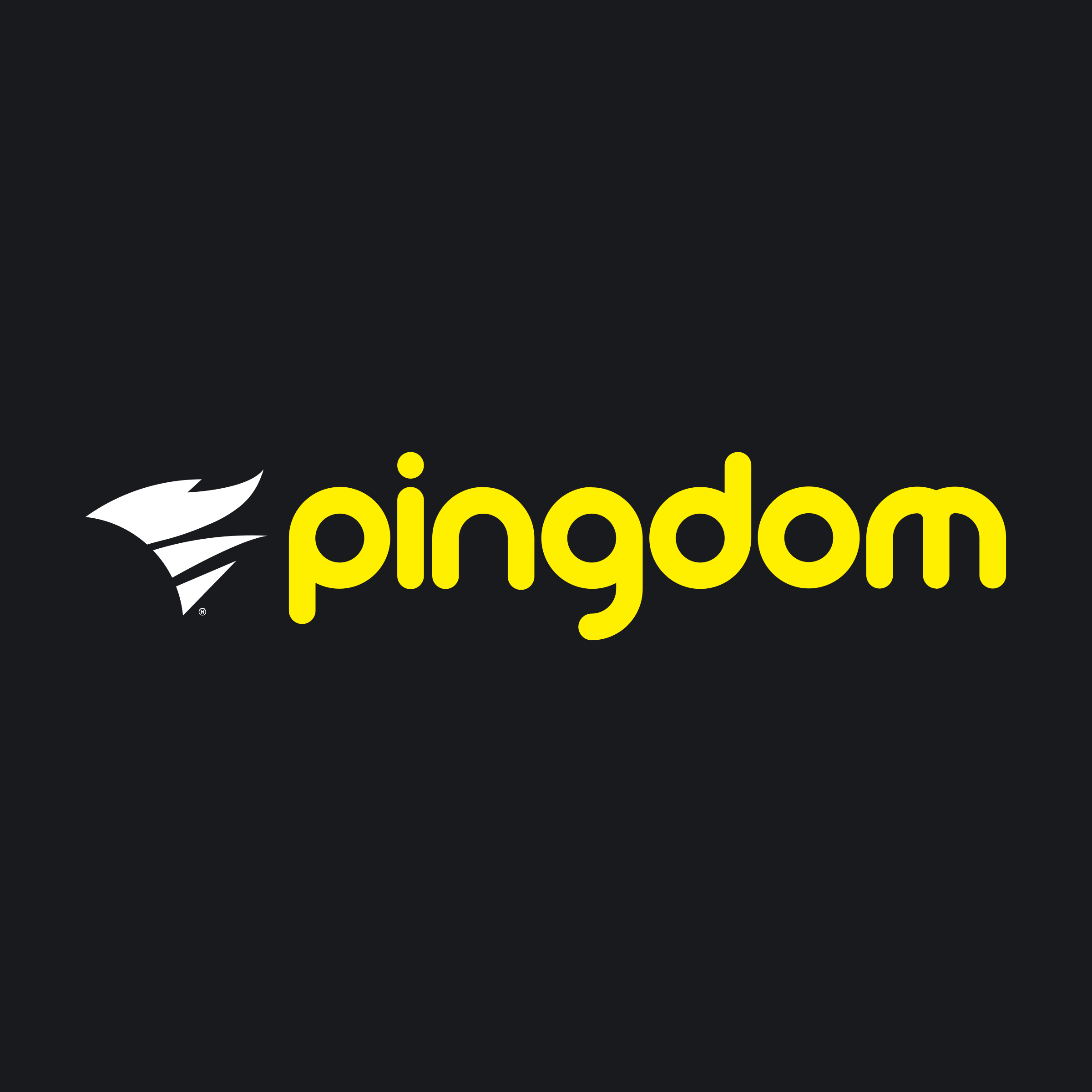
pingdom
DevelopmentThe Pingdom API is a way for you to automate your interaction with the Pingdom system. With the API, you can create your own scripts or applications with most of the functionality you can find inside the Pingdom control panel.
π Documentation & Examples
Everything you need to integrate with pingdom
π Quick Start Examples
// pingdom API Example
const response = await fetch('https://www.pingdom.com/api/2.1/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Pingdom Public API 2.1 - A Comprehensive Guide
Pingdom Public API 2.1 provides a powerful interface to automate, integrate and extend Pingdom's monitoring and alerting capabilities. This guide will give you a brief outline of Pingdom API and a few examples of how the API can be used in JavaScript.
Authentication
Before using the Pingdom API, the user needs to authenticate through the user's Pingdom account using API tokens.
API Endpoints
Pingdom Public API 2.1 supports the following endpoints:
- Checks
- Contacts
- Probes
- Raw Data
- Maintenance Windows
- Teams
- Integrations
JavaScript API Example Codes
Here are a few examples of how to use Pingdom API in JavaScript:
Example 1: Get a list of all checks
const Pingdom = require('pingdom-api');
const pingdom = new Pingdom({
user: 'YOUR_EMAIL',
pass: 'YOUR_PASSWORD',
token: 'YOUR_API_TOKEN',
});
pingdom.checks((err, res, body) => {
if (err) console.error(err);
console.log(body);
});
Example 2: Create a new check
pingdom.checks.create({
name: 'My Check',
host: 'example.com',
resolution: 5,
}, (err, res, body) => {
if (err) console.error(err);
console.log(body);
});
Example 3: Get a list of all contacts
pingdom.contacts((err, res, body) => {
if (err) console.error(err);
console.log(body);
});
Example 4: Create a new contact
pingdom.contacts.create({
name: 'My Contact',
type: 'email',
address: 'me@example.com',
}, (err, res, body) => {
if (err) console.error(err);
console.log(body);
});
Conclusion
Pingdom API provides powerful methods to get all the benefits of automated monitoring and alerting. The above-mentioned examples give you a brief introduction to how the JavaScript API can be used with the Pingdom Public API 2.1. To learn more about the API, visit the Pingdom API 2.1 documentation.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes