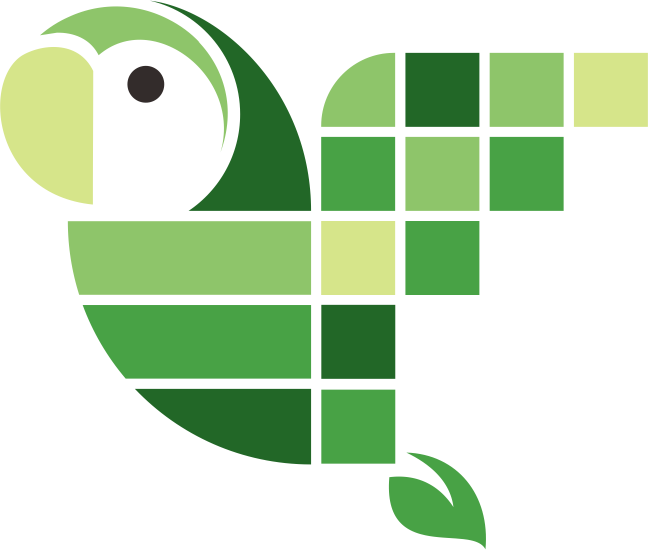
Pixela
Documents & ProductivityUsing the Pixe.la Public API with JavaScript
If you're looking for a way to track your habits or fitness goals, you might want to check out Pixe.la – a pixel art inspired app that allows you to create customizable graphs and track your progress over time. One of the coolest things about it is that it also has a public API that you can use to interact with the service programmatically. In this tutorial, we'll cover how to get started with the Pixe.la API using JavaScript.
1. Authentication
Before we can make requests to the Pixe.la API, we need to authenticate. Pixe.la uses Basic Authentication, which means we need to include our username and a special token in the request headers.
Here's an example of how to authenticate with JavaScript:
const username = 'your_username'
const token = 'your_token'
const authHeader = 'Basic ' + btoa(username + ':' + token)
fetch('https://pixe.la/v1/users/your_username', {
headers: {
'Authorization': authHeader
}
})
.then(response => {
console.log(response.status)
})
.catch(error => {
console.error(error)
})
In this example, we're using the fetch
API to make a GET request to our user profile page. We include the Authorization
header with our encoded credentials using the btoa
function. If everything goes well, we should see a 200 OK
status code in the console.
2. Creating Pixels
Now that we're authenticated, we can start creating pixels – single data points that represent the value of a habit at a specific date. Here's an example of how to create a pixel with JavaScript:
const graphID = 'your_graph_id'
const date = '20220101'
const quantity = '5'
fetch('https://pixe.la/v1/users/your_username/graphs/' + graphID, {
method: 'POST',
headers: {
'Authorization': authHeader,
'Content-Type': 'application/json'
},
body: JSON.stringify({
date: date,
quantity: quantity
})
})
.then(response => {
console.log(response.status)
})
.catch(error => {
console.error(error)
})
To create a pixel, we first need to know the graphID
of the graph we want to add it to. This can be found in the graph's URL or by making a GET request to /v1/users/your_username/graphs
and looking at the id
field of the graph we want to edit.
We then make a POST request to the /v1/users/your_username/graphs/your_graph_id
endpoint, including the date
and quantity
properties of the pixel we want to create in the request body. Again, we include the Authorization
header with our encoded credentials.
If our request is successful, we should see a 201 Created
status code in the console.
3. Updating Pixels
If we've made a mistake or want to update the value of a pixel, we can use the PUT method to change its quantity
property. Here's an example of how to update a pixel with JavaScript:
const graphID = 'your_graph_id'
const date = '20220101'
const quantity = '10'
fetch('https://pixe.la/v1/users/your_username/graphs/' + graphID + '/' + date, {
method: 'PUT',
headers: {
'Authorization': authHeader,
'Content-Type': 'application/json'
},
body: JSON.stringify({
quantity: quantity
})
})
.then(response => {
console.log(response.status)
})
.catch(error => {
console.error(error)
})
Here, we're making a PUT request to /v1/users/your_username/graphs/your_graph_id/20220101
, including the new quantity
value in the request body. If successful, we should see a 200 OK
status code in the console.
4. Removing Pixels
Finally, if we no longer need a pixel, we can use the DELETE method to remove it from the graph. Here's an example of how to delete a pixel with JavaScript:
const graphID = 'your_graph_id'
const date = '20220101'
fetch('https://pixe.la/v1/users/your_username/graphs/' + graphID + '/' + date, {
method: 'DELETE',
headers: {
'Authorization': authHeader,
}
})
.then(response => {
console.log(response.status)
})
.catch(error => {
console.error(error)
})
This time, we're making a DELETE request to /v1/users/your_username/graphs/your_graph_id/20220101
, without a request body. If successful, we should see a 204 No Content
status code in the console.
Conclusion
Using the Pixe.la public API with JavaScript is a great way to interact with the service programmatically and automate habit tracking. In this tutorial, we covered how to authenticate, create, update, and delete pixels using the fetch
API. As always, make sure to read the official documentation for more information and examples.