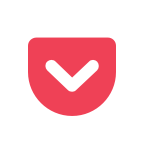
Getpocket API Docs
Are you looking for a simple way to integrate Pocket's features into your application? Look no further than the Getpocket API. Below we've provided some examples of how to use the API in JavaScript.
Authentication
Before you can interact with Pocket data, you'll need to authenticate to the service. The following code shows how to obtain a request token, which can be used with Pocket's OAuth flow:
fetch("https://getpocket.com/v3/oauth/request", {
method: "POST",
headers: {
"Content-Type": "application/json",
"X-Accept": "application/json"
},
body: JSON.stringify({
consumer_key: "[your consumer key here]",
redirect_uri: "[your redirect URI here]"
})
}).then(response => {
return response.json();
}).then(data => {
// Store or display the returned request token
console.log(data.code);
});
Adding an Item
Once you have an authorized user, you can add items to their Pocket account with ease. Here's an example of adding an article to their list:
fetch("https://getpocket.com/v3/add", {
method: "POST",
headers: {
"Content-Type": "application/json",
"X-Accept": "application/json"
},
body: JSON.stringify({
consumer_key: "[your consumer key here]",
access_token: "[user access token here]",
url: "[article URL here]"
})
}).then(response => {
return response.json();
}).then(data => {
// Store or display the created item's ID
console.log(data.item_id);
});
Getting Items
Retrieving items from a user's Pocket account is also straightforward. Here's a code snippet that shows how to get all unread items:
fetch("https://getpocket.com/v3/get", {
method: "POST",
headers: {
"Content-Type": "application/json",
"X-Accept": "application/json"
},
body: JSON.stringify({
consumer_key: "[your consumer key here]",
access_token: "[user access token here]",
state: "unread",
sort: "newest",
count: 10
})
}).then(response => {
return response.json();
}).then(data => {
// Do something with the retrieved items
console.log(data.list);
});
Conclusion
The Getpocket API provides a simple interface to interact with Pocket's powerful features. With the examples above, you can easily authenticate users, add items to their accounts, and retrieve data. Get started with Pocket development today!